void BinTreeDestroy(TNODE **root)用这个函数名写一个二叉树的销毁C语言
时间: 2023-08-10 11:05:17 浏览: 96
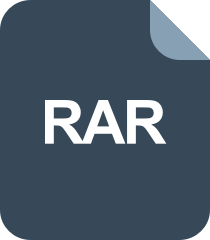
基于C语言的数据结构-二叉搜索树bitTree
以下是一个简单的二叉树销毁的 C 语言代码:
```c
typedef struct TreeNode {
int data;
struct TreeNode *left;
struct TreeNode *right;
} TNODE;
void BinTreeDestroy(TNODE **root) {
if (*root == NULL) {
return;
}
BinTreeDestroy(&((*root) -> left));
BinTreeDestroy(&((*root) -> right));
free(*root);
*root = NULL;
}
```
在这个函数中,我们首先检查二叉树的根节点是否为空。如果是空的,那么我们什么也不做,直接返回。否则,我们递归地销毁左子树和右子树,最后释放根节点并将其设置为 NULL。这可以确保我们不会意外地访问已经释放的内存。
阅读全文
相关推荐















