用c语言写一个根据后序和中序遍历输出先序遍历的程序
时间: 2023-03-13 13:29:34 浏览: 87
我可以为您提供一个示例程序,以便您参考: #include<stdio.h>
/* A binary tree tNode has data, pointer to left child
and a pointer to right child */
struct tNode
{
int data;
struct tNode* left;
struct tNode* right;
};
/* Function to traverse the binary tree in preorder */
void preorder(struct tNode* root)
{
if (root == NULL)
return;
/* first print data of tNode */
printf("%d ", root->data);
/* then recur on left sutree */
preorder(root->left);
/* now recur on right subtree */
preorder(root->right);
}
/* UTILITY FUNCTIONS */
/* Helper function that allocates a new tNode with the
given data and NULL left and right pointers. */
struct tNode* newtNode(int data)
{
struct tNode* tNode = (struct tNode*)
malloc(sizeof(struct tNode));
tNode->data = data;
tNode->left = NULL;
tNode->right = NULL;
return(tNode);
}
/* Driver program to test above functions*/
int main()
{
/* Constructed binary tree is
1
/ \
2 3
/ \
4 5
*/
struct tNode *root = newtNode(1);
root->left = newtNode(2);
root->right = newtNode(3);
root->left->left = newtNode(4);
root->left->right = newtNode(5);
preorder(root);
return 0;
}
阅读全文
相关推荐
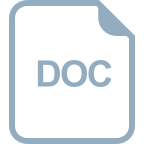
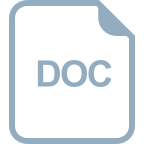
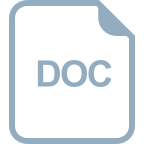

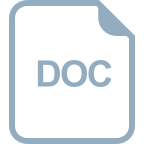



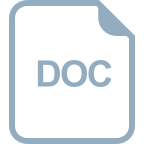









