用c语言写一个用后序和中序遍历输出先序遍历的程序
时间: 2023-03-13 17:30:46 浏览: 86
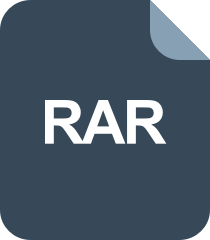
实现先序,中序和后序遍历的二叉树遍历程序

我可以为你提供一个示例程序:
#include <stdio.h>
/* 二叉树的节点定义 */
struct node
{
int data;
struct node *lchild;
struct node *rchild;
};
/* 先序遍历:先访问根节点,再访问左子树,最后访问右子树 */
void preOrder(struct node *root)
{
if(root != NULL)
{
printf("%d ", root->data);
preOrder(root->lchild);
preOrder(root->rchild);
}
}
/* 中序遍历:先访问左子树,再访问根节点,最后访问右子树 */
void inOrder(struct node *root)
{
if(root != NULL)
{
inOrder(root->lchild);
printf("%d ", root->data);
inOrder(root->rchild);
}
}
/* 后序遍历:先访问左子树,再访问右子树,最后访问根节点 */
void postOrder(struct node *root)
{
if(root != NULL)
{
postOrder(root->lchild);
postOrder(root->rchild);
printf("%d ", root->data);
}
}
/* 主函数 */
int main()
{
/* 假设二叉树如下:
1
/ \
2 3
/ \ / \
4 5 6 7
*/
struct node n1,n2,n3,n4,n5,n6,n7;
n1.data = 1;
n1.lchild = &n2;
n1.rchild = &n3;
n2.data = 2;
n2.lchild = &n4;
n2.rchild = &n5;
n3.data = 3;
n3.lchild = &n6;
n3.rchild = &n7;
n4.data = 4;
n4.lchild = NULL;
n4.rchild = NULL;
n5.data = 5;
n5.lchild = NULL;
n5.rchild = NULL;
n6.data = 6;
n6.lchild = NULL;
n6.rchild = NULL;
n7.data = 7;
n7.lchild = NULL;
n7.rchild = NULL;
printf("先序遍历:");
preOrder(&n1);
printf("\n");
printf("中序遍历:");
inOrder(&n1);
printf("\n");
printf("后序遍历:");
postOrder(&n1);
printf("\n");
return 0;
}
阅读全文
相关推荐









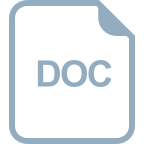

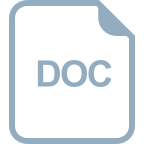
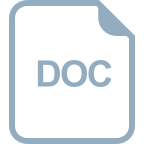


