springboot 视频上传下载
时间: 2023-10-19 08:10:04 浏览: 92
作为 AI 语言模型,我无法提供视频演示。不过,下面是 Spring Boot 实现视频上传下载的基本步骤:
1. 依赖配置
首先,需要在 pom.xml 文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
```
2. 文件上传
在 Spring Boot 中,可以使用 MultipartFile 类型接收上传的文件。在控制器中,可以使用 @RequestParam 注解来获取文件,并使用 FileUtils 类来保存文件。
```
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) throws IOException {
String fileName = file.getOriginalFilename();
File dest = new File("upload/" + fileName);
FileUtils.copyInputStreamToFile(file.getInputStream(), dest);
return "upload success";
}
```
3. 文件下载
在 Spring Boot 中,可以使用 ResponseEntity 类型来返回文件。在控制器中,使用以下代码来返回文件:
```
@GetMapping("/download")
public ResponseEntity<Resource> downloadFile() throws IOException {
File file = new File("upload/video.mp4");
InputStreamResource resource = new InputStreamResource(new FileInputStream(file));
HttpHeaders headers = new HttpHeaders();
headers.add(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=" + file.getName());
return ResponseEntity.ok()
.headers(headers)
.contentLength(file.length())
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(resource);
}
```
以上就是 Spring Boot 实现视频上传下载的基本步骤。
阅读全文
相关推荐




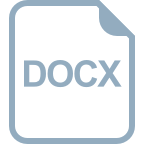
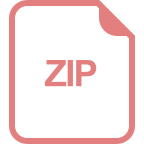
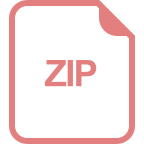








