51单片机DS1302时钟模块使用代码
时间: 2023-09-17 11:08:48 浏览: 122
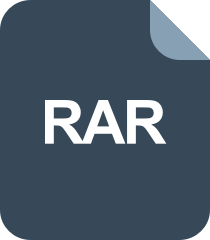
51单片机+LCD1602+DS1302组成的电子时钟电路包含源代码和Proteus仿真电路

以下是一个基于51单片机的DS1302时钟模块使用代码示例:
```c
#include <REG52.h>
#include <intrins.h>
#define uchar unsigned char
#define uint unsigned int
sbit RST = P3^4;
sbit DAT = P3^5;
sbit CLK = P3^6;
// 延时函数
void Delay(uint i)
{
while(i--);
}
// 向DS1302写入一个字节
void DS1302_WriteByte(uchar dat)
{
uchar i;
for (i = 0; i < 8; i++)
{
DAT = dat & 0x01;
CLK = 0;
CLK = 1;
dat >>= 1;
}
}
// 从DS1302读取一个字节
uchar DS1302_ReadByte()
{
uchar i, dat = 0;
for (i = 0; i < 8; i++)
{
dat >>= 1;
if (DAT)
dat |= 0x80;
CLK = 0;
CLK = 1;
}
return dat;
}
// 启动DS1302时钟
void DS1302_Start()
{
RST = 1;
CLK = 1;
_nop_();
_nop_();
_nop_();
RST = 0;
DS1302_WriteByte(0x8e);
DS1302_WriteByte(0x00);
RST = 1;
}
// 停止DS1302时钟
void DS1302_Stop()
{
RST = 0;
CLK = 0;
_nop_();
_nop_();
_nop_();
RST = 1;
DS1302_WriteByte(0x8e);
DS1302_WriteByte(0x80);
RST = 1;
}
// 写入DS1302时间
void DS1302_WriteTime(uchar year, uchar month, uchar day, uchar hour, uchar minute, uchar second)
{
DS1302_Start();
DS1302_WriteByte(0xbe);
DS1302_WriteByte(second);
DS1302_WriteByte(minute);
DS1302_WriteByte(hour);
DS1302_WriteByte(day);
DS1302_WriteByte(month);
DS1302_WriteByte(year);
DS1302_Stop();
}
// 读取DS1302时间
void DS1302_ReadTime(uchar *year, uchar *month, uchar *day, uchar *hour, uchar *minute, uchar *second)
{
DS1302_Start();
DS1302_WriteByte(0xbf);
*second = DS1302_ReadByte();
*minute = DS1302_ReadByte();
*hour = DS1302_ReadByte();
*day = DS1302_ReadByte();
*month = DS1302_ReadByte();
*year = DS1302_ReadByte();
DS1302_Stop();
}
void main()
{
uchar year, month, day, hour, minute, second;
DS1302_WriteTime(0x21, 0x10, 0x13, 0x10, 0x30, 0x00);
while (1)
{
DS1302_ReadTime(&year, &month, &day, &hour, &minute, &second);
printf("20%d-%d-%d %d:%d:%d\n", year, month, day, hour, minute, second);
Delay(1000);
}
}
```
在上述代码中,定义了DS1302的RST、DAT、CLK引脚,以及自定义了延时函数`Delay`。然后定义了向DS1302写入一个字节的函数`DS1302_WriteByte`,从DS1302读取一个字节的函数`DS1302_ReadByte`,启动DS1302时钟的函数`DS1302_Start`,停止DS1302时钟的函数`DS1302_Stop`,写入DS1302时间的函数`DS1302_WriteTime`,以及读取DS1302时间的函数`DS1302_ReadTime`。
在`main`函数中,首先调用`DS1302_WriteTime`函数设置初始时间,然后通过`DS1302_ReadTime`函数读取当前时间,并通过串口输出。最后通过`Delay`函数延迟1秒,实现时钟的运行。需要注意的是,DS1302时钟模块的时间格式为BCD码,需要进行转换才能得到实际的时间。
阅读全文
相关推荐
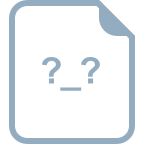
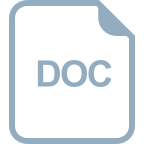
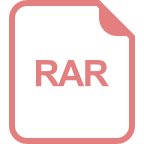
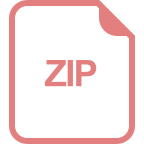
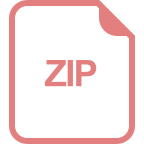
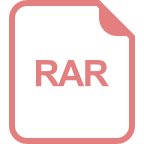
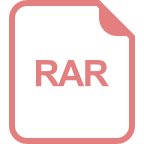
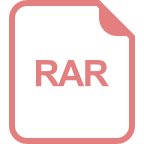
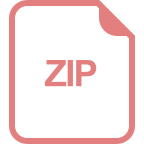
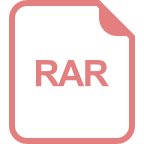
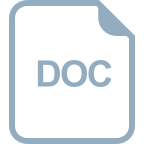
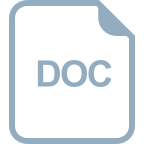
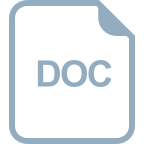
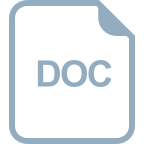
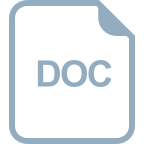

