CStdioFile::Open() 函数以“rt,ccs=UTF-8”模式打开文件
时间: 2024-04-08 12:35:44 浏览: 9
是的,你可以使用 CStdioFile::Open() 函数以 "rt,ccs=UTF-8" 模式打开文件,以确保以 UTF-8 编码方式读取文件内容。这样可以避免中文乱码问题。
以下是一个示例代码:
```cpp
CStdioFile file;
CString strLine;
if (file.Open(_T("file.txt"), CFile::modeRead | CFile::typeText, CStdioFile::shareDenyNone, NULL, _T("rt,ccs=UTF-8")))
{
while (file.ReadString(strLine))
{
// 处理读取到的每一行内容
// ...
}
file.Close();
}
```
在上述代码中,通过在 Open() 函数中传递 "rt,ccs=UTF-8" 参数,将文件以 UTF-8 编码方式打开,确保读取到的内容是正确的中文字符串。
请注意,确保文件实际上是以 UTF-8 编码保存的。如果文件不是 UTF-8 编码,可能会导致读取出来的内容仍然是乱码。如果文件编码方式不是 UTF-8,需要相应地调整打开文件的参数。
希望这可以帮助你在 MFC 中正确处理中文文件的读取。如果你有其他问题,请随时提问。
相关问题
MFC中以UTF-8编码格式读取txt文件
在 MFC 中以 UTF-8 编码格式读取 txt 文件你可以使用下面来实现:
```
CStdioFile file;
CString strLine;
if (file.Open(_T("file.txt"), CFile::modeRead | CFile::typeText | C::shareDenyNone, NULL, NULL, CStdioFile::typeUnicode))
{
CStringA strA;
while (file.ReadString(strA))
{
CStringW strW;
int length = MultiByteToWideChar(CP_UTF8, 0, strA, -1, NULL, 0);
MultiByteToWideChar(CP_UTF8, 0, strA, -1, strW.GetBuffer(length), length);
strW.ReleaseBuffer();
// 处理读取到的每一行内容(使用 Unicode 字符串 strW)
// ...
}
file.Close();
}
```
在上述代码中,我们使用了 CStdioFile::Open() 函数以 `CFile::typeText` 和 `CStdioFile::typeUnicode` 参数打开文件。这样可以确保以 Unicode 格式读取文件内容。
然后,在循环中,我们使用了 MultiByteToWideChar() 函数将 UTF-8 编码的字符串转换为 Unicode 字符串。这样可以正确处理 UTF-8 编码的中文内容。
请注意,如果你的文件内容不是以 UTF-8 编码保存的,那么上述代码可能会导致乱码。确保文件实际上是以 UTF-8 编码保存的。
希望这可以帮助你在 MFC 中以 UTF-8 编码格式读取 txt 文件。如果你有其他问题,请随时提问。
在多字截环境下用cstdiofile读取utf-8的txt文件,分割显示到listctrl控件中,要求不出现中文乱码且至少四列四行
好的,我明白了您的问题。以下是我给出的解决方案:
1. 首先,您需要使用 Unicode 编码来读取 UTF-8 文件,因为 UTF-8 使用的是多字节编码。您可以使用 `wifstream` 来打开文件,使用 `wstringstream` 来读取文件内容,并使用 `wstring` 存储读取的字符串。
2. 接下来,您需要将读取到的字符串按照您的需求进行分割。您可以使用 `wistringstream` 将字符串分割成单独的项,并逐个添加到您的 `listctrl` 控件中。
3. 为了避免中文乱码,您需要将您的程序的默认编码设置为 UTF-8。您可以使用以下代码来设置:
```
setlocale(LC_ALL, "en_US.UTF-8");
```
4. 最后,根据您的要求,您需要使用 `listctrl` 控件的 `InsertColumn` 和 `InsertItem` 方法来创建至少四列四行的表格,并将分割后的项逐个添加到表格中。
下面是一个完整的示例代码,您可以参考一下:
```c++
#include <fstream>
#include <sstream>
#include <vector>
#include <iostream>
#include <locale.h>
#include <afxwin.h>
using namespace std;
int main()
{
// 设置默认编码为 UTF-8
setlocale(LC_ALL, "en_US.UTF-8");
// 打开文件
wifstream file("test.txt");
// 读取文件内容
wstringstream buffer;
buffer << file.rdbuf();
wstring content = buffer.str();
// 分割字符串
vector<wstring> items;
wistringstream iss(content);
wstring item;
while (getline(iss, item, L'\t')) {
items.push_back(item);
}
// 创建表格
CListCtrl listctrl;
listctrl.Create(WS_VISIBLE | WS_BORDER | LVS_REPORT, CRect(10, 10, 300, 200), NULL, NULL);
listctrl.InsertColumn(0, L"列1", LVCFMT_LEFT, 100);
listctrl.InsertColumn(1, L"列2", LVCFMT_LEFT, 100);
listctrl.InsertColumn(2, L"列3", LVCFMT_LEFT, 100);
listctrl.InsertColumn(3, L"列4", LVCFMT_LEFT, 100);
// 添加数据
int row = 0;
for (int i = 0; i < 16; i += 4) {
listctrl.InsertItem(row, items[i].c_str());
listctrl.SetItemText(row, 1, items[i + 1].c_str());
listctrl.SetItemText(row, 2, items[i + 2].c_str());
listctrl.SetItemText(row, 3, items[i + 3].c_str());
row++;
}
return 0;
}
```
注意:由于我不知道您的具体环境和要求,以上代码可能需要进行一些修改才能正常运行。
相关推荐
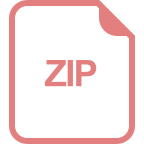













