用指针编程实现一个字符统计程序要求输入最多200个字符的字符串输出字符总个数大写字母个数小写字母个数数字个数以及其他字符的个数
时间: 2024-01-26 11:04:55 浏览: 180
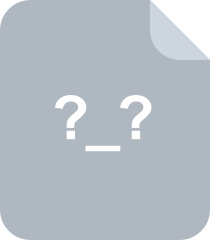
输入一个字符串,统计出这个字符串的英文大写字母、小写字母、数字、空格以及其它字符的个数

下面是一个用指针编程实现字符统计程序的示例代码:
```c
#include <stdio.h>
#include <ctype.h>
#define MAXLEN 200
void count_chars(char *str, int *total, int *upper, int *lower, int *digit, int *other);
int main() {
char str[MAXLEN + 1];
int total, upper, lower, digit, other;
printf("请输入一个最多包含%d个字符的字符串:\n", MAXLEN);
fgets(str, MAXLEN + 1, stdin);
count_chars(str, &total, &upper, &lower, &digit, &other);
printf("字符串中共有%d个字符,其中:\n", total);
printf("大写字母:%d个\n", upper);
printf("小写字母:%d个\n", lower);
printf("数字:%d个\n", digit);
printf("其他字符:%d个\n", other);
return 0;
}
void count_chars(char *str, int *total, int *upper, int *lower, int *digit, int *other) {
*total = *upper = *lower = *digit = *other = 0;
while (*str != '\0' && *str != '\n') {
(*total)++;
if (isupper(*str))
(*upper)++;
else if (islower(*str))
(*lower)++;
else if (isdigit(*str))
(*digit)++;
else
(*other)++;
str++;
}
}
```
在该程序中,使用了 `count_chars` 函数来统计字符个数,并将统计结果通过指针返回给主函数。在 `count_chars` 函数中,使用了指针来修改传入的参数的值,从而实现了字符统计的功能。
阅读全文
相关推荐
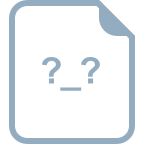
















