设计一个Rectangle类,定义两个double属性分别表示宽高默认为1并用GetArea返回矩形面积
时间: 2024-05-13 10:15:35 浏览: 93
下面是一个简单的Rectangle类的实现,其中包含两个double类型的属性width和height,以及一个GetArea方法,用于返回矩形的面积。
```csharp
public class Rectangle
{
private double width = 1;
private double height = 1;
public double Width
{
get { return width; }
set { width = value; }
}
public double Height
{
get { return height; }
set { height = value; }
}
public double GetArea()
{
return width * height;
}
}
```
使用方法:
```csharp
Rectangle rect = new Rectangle();
Console.WriteLine(rect.GetArea()); // 输出 1
rect.Width = 5;
rect.Height = 10;
Console.WriteLine(rect.GetArea()); // 输出 50
```
相关问题
设计一个Rectangle类,定义两个double属性并用GetArea
来计算其面积。
好的,以下是一个简单的Rectangle类的示例:
```python
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def get_area(self):
return self.width * self.height
```
这个类有一个初始化方法,它接受两个参数:width和height。这些参数分别初始化了类的width和height属性。这个类还有一个get_area方法,它返回width和height属性的乘积,即矩形的面积。你可以使用以下代码测试该类的功能:
```python
r = Rectangle(5, 10)
print("矩形的面积为:", r.get_area())
```
输出结果为:
```
矩形的面积为: 50
```
设计一个名为 Rectangle 的类表示矩形 定义两个属性: double width 和 double height 分别表示宽和高,默认都为 1 创建默认举行的无参构造方法 使用指定宽和高创建矩形对象的构造方法 一个名为 getArea 的返回矩形
的面积的方法,公式为面积 = 宽 * 高,一个名为 getPerimeter 的返回矩形的周长的方法,公式为周长 = 2 * (宽 + 高)。
下面是实现该类的示例代码:
```java
public class Rectangle {
private double width;
private double height;
public Rectangle() {
this.width = 1;
this.height = 1;
}
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double getArea() {
return this.width * this.height;
}
public double getPerimeter() {
return 2 * (this.width + this.height);
}
}
```
可以通过以下方式创建并使用矩形对象:
```java
Rectangle defaultRectangle = new Rectangle(); // 宽和高都为 1 的默认矩形
Rectangle customRectangle = new Rectangle(3, 4); // 宽为 3,高为 4 的自定义矩形
double area = defaultRectangle.getArea(); // 获取默认矩形的面积
double perimeter = customRectangle.getPerimeter(); // 获取自定义矩形的周长
```
阅读全文
相关推荐
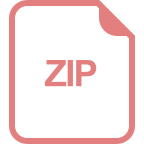
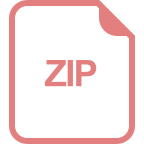
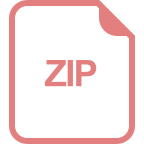













