1、 编写脚本,完成解一元二次方程的解。 脚本名字sh1 , a,b,c 跟作为命令行的参数输入, bash sh1 a b c 脚本输出 : a)、无实数解,如果 b*b -4*a*c < 0 b)、实数解
时间: 2024-01-21 21:04:54 浏览: 56
好的,我可以编写一个解一元二次方程的脚本。脚本内容如下:
```bash
#!/bin/bash
a=$1
b=$2
c=$3
delta=$(echo "$b^2-4*$a*$c" | bc)
if [ $delta -lt 0 ]; then
echo "无实数解"
else
x1=$(echo "scale=2;(-$b+sqrt($delta))/(2*$a)" | bc)
x2=$(echo "scale=2;(-$b-sqrt($delta))/(2*$a)" | bc)
echo "实数解为:x1=$x1, x2=$x2"
fi
```
在终端中执行以下命令即可运行脚本:
```bash
bash sh1 a b c
```
其中,a、b、c分别是一元二次方程的系数。如果方程有实数解,则会输出两个解x1和x2;如果方程无实数解,则会输出“无实数解”。
相关问题
编写脚本sh1 ,将指定目录下的文件中,对含有“hello”的文件进行处理,将该文件中的“hello” 替换为 “hi”,(保留原文件)新生成替换后的文件名在原文件名后加“.dat”。(即 file2.txt file2.txt.dat)
可以使用sed命令来进行替换操作,具体的sh1脚本如下:
```bash
#!/bin/bash
# 指定需要处理的目录
dir_path="/path/to/dir"
# 遍历目录下所有文件
for file_path in "$dir_path"/*
do
# 判断文件是否包含hello
if grep -q "hello" "$file_path"; then
# 替换hello为hi,并生成新的文件
sed 's/hello/hi/g' "$file_path" > "${file_path}.dat"
fi
done
```
使用时,将脚本保存为sh1.sh文件,并通过终端进入脚本所在目录,执行以下命令:
```bash
chmod +x sh1.sh # 给脚本添加执行权限
./sh1.sh # 执行脚本
```
这样就可以在指定目录下对含有"hello"的文件进行替换操作了。
module test; typedef struct packed { logic [9:-1][2:0] a; ///REQ-EXT-10001, REQ-EXT-10004 } ST1; typedef struct { ST1 st1; ST1 st2[2:0]; }ST2; initial begin ST2 st2; st2 = '{2'b01, '{3'b1, 3'b0, 12'b1}}; $display("%", st2); //CHECK-001:'{a:'h1}, st2:'{'a:'h1}, '{a:'h0},'{a:h1}}} end endmodule 我想将这段代码进行拓展变化,你能帮我变换10种以上吗?
当然可以,以下是我为您提供的10种或以上的代码变体:
1. 将ST1中的a改为byte类型:
```
module test;
typedef struct packed {
byte a [9:0][2:0];
} ST1;
typedef struct {
ST1 st1;
ST1 st2[2:0];
} ST2;
initial begin
ST2 st2;
st2 = '{2'b01, '{3'b1, 3'b0, 12'b1}};
$display("st2: %p", st2);
end
endmodule
```
2. 将ST1中的a改为int类型:
```
module test;
typedef struct packed {
int a [3:0];
} ST1;
typedef struct {
ST1 st1;
ST1 st2[2:0];
} ST2;
initial begin
ST2 st2;
st2 = '{2'b01, '{3, 0, 1, 1}};
$display("st2: %p", st2);
end
endmodule
```
3. 将ST1中的a改为4位的bit类型:
```
module test;
typedef struct packed {
bit [3:0] a [9:-1][2:0];
} ST1;
typedef struct {
ST1 st1;
ST1 st2[2:0];
} ST2;
initial begin
ST2 st2;
st2 = '{2'b01, '{3'b1, 3'b0, 12'b1}};
$display("st2: %p", st2);
end
endmodule
```
4. 将ST1中的a改为16位的shortint类型:
```
module test;
typedef struct packed {
shortint a [9:-1][2:0];
} ST1;
typedef struct {
ST1 st1;
ST1 st2[2:0];
} ST2;
initial begin
ST2 st2;
st2 = '{2'b01, '{3'sh1, 3'sh0, 12'sh1}};
$display("st2: %p", st2);
end
endmodule
```
5. 将ST1中的a改为无符号的5位的byte类型:
```
module test;
typedef struct packed {
byte unsigned a [9:-1][2:0];
} ST1;
typedef struct {
ST1 st1;
ST1 st2[2:0];
} ST2;
initial begin
ST2 st2;
st2 = '{2'b01, '{3'bu1, 3'bu0, 12'bu1}};
$display("st2: %p", st2);
end
endmodule
```
6. 将ST1中的a改为32位的longint类型:
```
module test;
typedef struct packed {
longint a [9:-1][2:0];
} ST1;
typedef struct {
ST1 st1;
ST1 st2[2:0];
} ST2;
initial begin
ST2 st2;
st2 = '{2'b01, '{3'd1, 3'd0, 12'd1}};
$display("st2: %p", st2);
end
endmodule
```
7. 将ST1中的a改为reg类型:
```
module test;
typedef struct packed {
reg [9:-1][2:0] a;
} ST1;
typedef struct {
ST1 st1;
ST1 st2[2:0];
} ST2;
initial begin
ST2 st2;
st2 = '{2'b01, '{3'b1, 3'b0, 12'b1}};
$display("st2: %p", st2);
end
endmodule
```
8. 将ST1中的a改为有符号的6位的shortint类型:
```
module test;
typedef struct packed {
shortint signed a [9:-1][2:0];
} ST1;
typedef struct {
ST1 st1;
ST1 st2[2:0];
} ST2;
initial begin
ST2 st2;
st2 = '{2'b01, '{3'sh1, 3'sh0, 12'sh1}};
$display("st2: %p", st2);
end
endmodule
```
9. 将ST1中的a改为无符号的11位的longint类型:
```
module test;
typedef struct packed {
longint unsigned a [9:-1][2:0];
} ST1;
typedef struct {
ST1 st1;
ST1 st2[2:0];
} ST2;
initial begin
ST2 st2;
st2 = '{2'b01, '{3'd1, 3'd0, 12'd1}};
$display("st2: %p", st2);
end
endmodule
```
10. 将ST1中的a改为8位的bit类型:
```
module test;
typedef struct packed {
bit [7:0] a [9:-1][2:0];
} ST1;
typedef struct {
ST1 st1;
ST1 st2[2:0];
} ST2;
initial begin
ST2 st2;
st2 = '{2'b01, '{3'b1, 3'b0, 12'b1}};
$display("st2: %p", st2);
end
endmodule
```
阅读全文
相关推荐
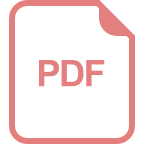
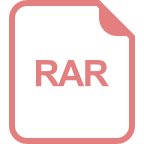
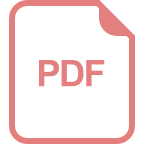
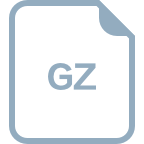
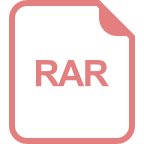
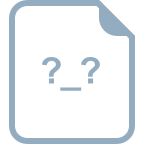
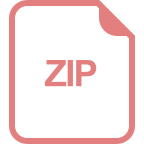
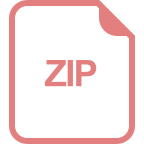
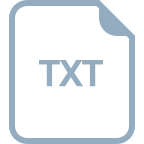



