输入N个英文单词,建立字符串数组,按字典顺序输出这些英文单词。要求用c语言指针
时间: 2023-09-16 07:04:57 浏览: 234
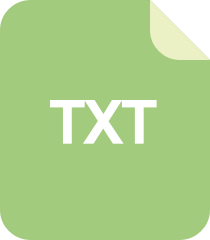
使用指针、数组处理在C语言中处理“字符串”
### 回答1:
你好,下面是使用 C 语言指针建立字符串数组并按字典序输出英文单词的代码示例:
```
#include <stdio.h>
#include <string.h>
#define MAX_WORDS 100
int main(void) {
// 定义字符串数组,并输入 N 个英文单词
char *words[MAX_WORDS];
int n;
printf("请输入单词个数:");
scanf("%d", &n);
printf("请输入 %d 个英文单词:\n", n);
for (int i = 0; i < n; i++) {
// 使用指针动态分配内存,并输入单词
char word[50];
scanf("%s", word);
words[i] = malloc(strlen(word) + 1);
strcpy(words[i], word);
}
// 使用冒泡排序将单词按字典序排序
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (strcmp(words[j], words[j + 1]) > 0) {
// 交换单词
char *temp = words[j];
words[j] = words[j + 1];
words[j + 1] = temp;
}
}
}
// 输出排序后的单词
printf("按字典序排序后的单词:\n");
for (int i = 0; i < n; i++) {
printf("%s\n", words[i]);
}
// 释放内存
for (int i = 0; i < n; i++) {
free(words[i]);
}
return 0;
}
```
上述代码使用了 C 语言的指针动态分配内存来建立字符串数组,并使用冒泡排序将单词按字典序排序。最后,通过循环输出排序后的单
### 回答2:
可以使用C语言指针来完成这个任务。首先,我们需要使用malloc函数来为字符串数组分配内存空间。假设我们输入的N个英文单词存储在一个名为words的数组中。
1. 首先,我们需要找到这N个英文单词中的最长单词的长度,以便为字符串数组分配足够的内存空间。
```c
int maxLength = 0;
for (int i = 0; i < N; i++) {
int len = strlen(words[i]);
if (len > maxLength) {
maxLength = len;
}
}
```
2. 接下来,我们使用malloc函数为字符串数组分配内存空间,并将每个单词复制到对应的数组元素中。
```c
char **sortedWords = (char **)malloc(N * sizeof(char *));
for (int i = 0; i < N; i++) {
sortedWords[i] = (char *)malloc((maxLength + 1) * sizeof(char));
strcpy(sortedWords[i], words[i]);
}
```
3. 然后,我们使用冒泡排序算法对字符串数组进行排序。
```c
for (int i = 0; i < N - 1; i++) {
for (int j = 0; j < N - i - 1; j++) {
if (strcmp(sortedWords[j], sortedWords[j + 1]) > 0) {
char *temp = sortedWords[j];
sortedWords[j] = sortedWords[j + 1];
sortedWords[j + 1] = temp;
}
}
}
```
4. 最后,我们按照字典顺序输出排序后的字符串数组。
```c
for (int i = 0; i < N; i++) {
printf("%s\n", sortedWords[i]);
}
```
请注意,完成排序后,我们需要逐个释放为字符串数组分配的内存空间。
```c
for (int i = 0; i < N; i++) {
free(sortedWords[i]);
}
free(sortedWords);
```
以上就是使用C语言指针来实现按字典顺序输出N个英文单词的方法。
### 回答3:
要实现上述需求,我们可以按照以下步骤进行:
1. 首先,定义一个字符串数组,用来存储输入的N个英文单词。假设数组名为words。
2. 使用一个循环,从用户输入中逐个读取N个英文单词,并将其存储到words数组中。
3. 定义一个临时指针变量temp,用于在排序过程中交换两个字符串的位置。
4. 使用嵌套的循环来遍历整个words数组,比较相邻两个字符串的字典顺序。如果前一个字符串大于后一个字符串,则交换它们的位置,以实现字典排序。
5. 重复上述步骤,直到整个数组按照字典顺序排列好。
6. 最后,使用一个循环遍历已排序的words数组,并逐个输出它们。
下面是示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
int N, i;
printf("请输入单词个数N:");
scanf("%d", &N);
// 声明字符串数组
char words[N][50];
// 逐个读取N个英文单词
for (i = 0; i < N; i++) {
printf("请输入第%d个单词:", i+1);
scanf("%s", words[i]);
}
// 对字符串数组进行字典排序
char *temp;
for (i = 0; i < N-1; i++) {
for (int j = 0; j < N-i-1; j++) {
if (strcmp(words[j], words[j+1]) > 0) {
temp = words[j];
words[j] = words[j+1];
words[j+1] = temp;
}
}
}
// 输出排序后的字符串数组
printf("按字典顺序排序后的单词为:");
for (i = 0; i < N; i++) {
printf("%s ", words[i]);
}
printf("\n");
return 0;
}
```
上述代码中,我们使用了字符串数组`words`来存储输入的英文单词,并通过`strcmp`函数来比较字符串的字典顺序。使用临时指针变量`temp`来进行交换操作,最终实现了按字典顺序输出单词的功能。
阅读全文
相关推荐



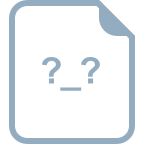

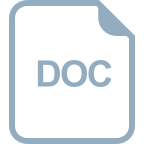
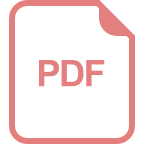
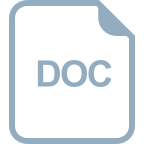
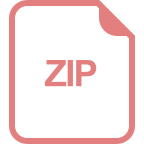
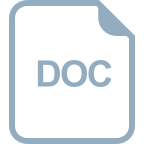
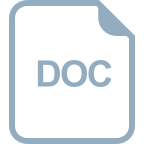
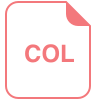
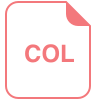
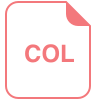
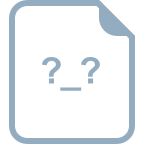