java http 接收xml参数_读写xml并使用xml文件进行参数传递给接口获取返回内容——wishPost请求物流单号为例...
时间: 2024-04-06 07:32:26 浏览: 63
首先,我们需要使用Java中的一个XML处理库,比如dom4j,来读写XML文件。接着,我们可以通过HTTP发送POST请求,并将XML参数作为请求体发送到接口。
以下是一个示例代码,演示了如何使用dom4j读取XML文件,并将XML参数作为请求体发送到API获取返回内容:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Iterator;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.io.SAXReader;
public class Main {
public static void main(String[] args) throws Exception {
// 读取XML文件
String xmlFilePath = "path/to/xml/file.xml";
SAXReader reader = new SAXReader();
Document document = reader.read(xmlFilePath);
// 获取XML参数
Element root = document.getRootElement();
String logisticsNo = root.elementText("logisticsNo");
String appKey = root.elementText("appKey");
String appSecret = root.elementText("appSecret");
// 发送HTTP请求
String apiUrl = "http://api.example.com/wishPost";
URL url = new URL(apiUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/xml");
// 设置请求体
String requestBody = "<request><logisticsNo>" + logisticsNo + "</logisticsNo><appKey>" + appKey + "</appKey><appSecret>" + appSecret + "</appSecret></request>";
conn.setDoOutput(true);
conn.getOutputStream().write(requestBody.getBytes("UTF-8"));
// 获取返回内容
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
StringBuilder stringBuilder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
stringBuilder.append(line);
}
reader.close();
String response = stringBuilder.toString();
System.out.println(response);
}
}
```
需要注意的是,这只是一个示例代码,需要根据实际情况进行修改和调整。
阅读全文
相关推荐


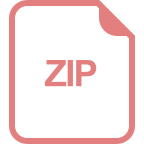






