用Java产生100个随机数并使用冒泡排序和选择排序对这些数进行排序
时间: 2023-05-27 15:06:50 浏览: 184
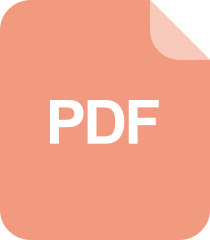
java利用冒泡排序对数组进行排序

以下是Java代码:
```java
import java.util.Arrays;
import java.util.Random;
public class Sort {
public static void main(String[] args) {
int[] arr = generateRandomArray(100);
System.out.println("Original array: " + Arrays.toString(arr));
int[] bubbleSortedArr = bubbleSort(arr.clone());
System.out.println("Bubble sorted array: " + Arrays.toString(bubbleSortedArr));
int[] selectionSortedArr = selectionSort(arr.clone());
System.out.println("Selection sorted array: " + Arrays.toString(selectionSortedArr));
}
// 产生指定长度的随机数组
public static int[] generateRandomArray(int length) {
int[] arr = new int[length];
Random random = new Random();
for (int i = 0; i < length; i++) {
arr[i] = random.nextInt(1000); // 产生0-999之间的随机数
}
return arr;
}
// 冒泡排序
public static int[] bubbleSort(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
for (int j = 0; j < arr.length - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
return arr;
}
// 选择排序
public static int[] selectionSort(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
if (minIndex != i) {
int temp = arr[i];
arr[i] = arr[minIndex];
arr[minIndex] = temp;
}
}
return arr;
}
}
```
阅读全文
相关推荐








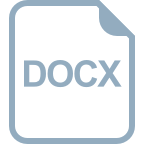





