c语言实现哈希表的查找
时间: 2023-09-22 18:12:11 浏览: 93
哈希表是一种常见的数据结构,通过哈希函数将关键字映射到表中的位置,实现快速的查找、插入和删除操作。下面是使用 C 语言实现哈希表的查找操作的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define HASH_TABLE_SIZE 100
typedef struct Node {
char key[20];
int value;
struct Node* next;
} Node;
Node* hash_table[HASH_TABLE_SIZE];
int hash_function(char* key) {
int sum = 0;
for (int i = 0; i < strlen(key); i++) {
sum += key[i];
}
return sum % HASH_TABLE_SIZE;
}
void insert(char* key, int value) {
int index = hash_function(key);
Node* new_node = (Node*)malloc(sizeof(Node));
strcpy(new_node->key, key);
new_node->value = value;
new_node->next = NULL;
if (hash_table[index] == NULL) {
hash_table[index] = new_node;
} else {
Node* node = hash_table[index];
while (node->next != NULL) {
node = node->next;
}
node->next = new_node;
}
}
int search(char* key) {
int index = hash_function(key);
Node* node = hash_table[index];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
return node->value;
}
node = node->next;
}
return -1;
}
int main() {
insert("apple", 10);
insert("banana", 20);
insert("orange", 30);
printf("%d\n", search("apple"));
printf("%d\n", search("banana"));
printf("%d\n", search("orange"));
printf("%d\n", search("watermelon"));
return 0;
}
```
在上面的代码中,我们定义了一个哈希表的大小为 100,使用字符串的 ASCII 码值之和作为哈希函数。在插入元素时,如果哈希表中对应的位置为空,则直接插入元素。否则,遍历该位置上的链表,将新元素插入到链表的末尾。在查找元素时,先根据关键字计算出哈希值,然后遍历该位置上的链表,查找目标元素。如果找到,则返回对应的值;如果没有找到,则返回 -1。
以上就是使用 C 语言实现哈希表的查找操作的示例代码,希望能够帮到你。
阅读全文
相关推荐
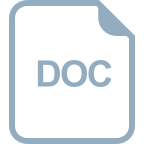
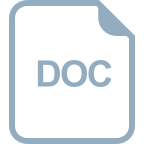
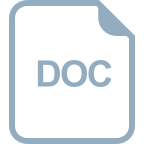
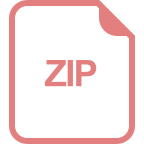
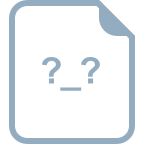
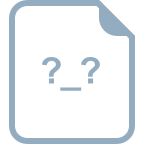
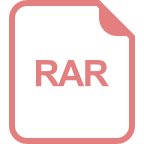
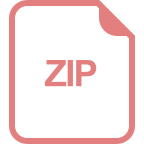
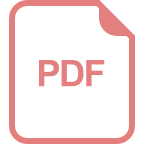
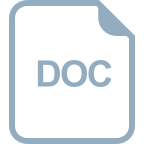
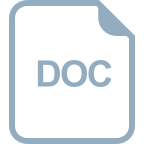
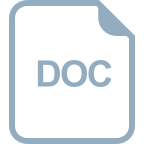
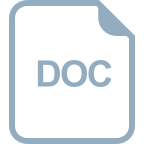
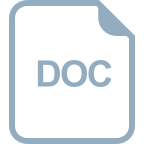
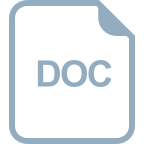
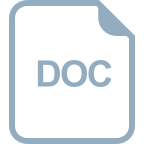
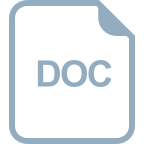
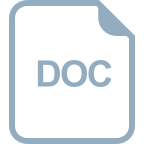
