二、遍历文件目录 //遍历dirPath目录树,先文件夹排序在前,文件在后,再按名称的sort排序 //dirPath:3~50个字符,不能包含特殊字符;sort:只能为["ASC","DESC"]顺序或逆序 void printDirTree(String dirPath,String sort); @Test public void testPrintDirTree();//测试void printDirTree(String dirPath,String sort)方法,须校验参数规则
时间: 2024-01-21 16:18:15 浏览: 72
好的,下面是一个可能符合要求的实现:
```
import java.io.File;
import java.util.Arrays;
import java.util.Comparator;
public class FileTreeTraverser {
public void printDirTree(String dirPath, String sort) {
// 校验参数规则
if (dirPath == null || dirPath.length() < 3 || dirPath.length() > 50 ||
!dirPath.matches("^[a-zA-Z0-9-_]+$")) {
throw new IllegalArgumentException("dirPath参数不符合规则");
}
if (!"ASC".equals(sort) && !"DESC".equals(sort)) {
throw new IllegalArgumentException("sort参数只能为ASC或DESC");
}
File root = new File(dirPath);
if (!root.isDirectory()) {
throw new IllegalArgumentException("dirPath不是目录");
}
// 先获取所有文件和文件夹
File[] files = root.listFiles();
// 对文件夹和文件排序,先文件夹在前,文件在后
Arrays.sort(files, new Comparator<File>() {
@Override
public int compare(File o1, File o2) {
if (o1.isDirectory() && !o2.isDirectory()) {
return -1;
} else if (!o1.isDirectory() && o2.isDirectory()) {
return 1;
} else {
return 0;
}
}
});
// 按名称排序
if ("ASC".equals(sort)) {
Arrays.sort(files, new Comparator<File>() {
@Override
public int compare(File o1, File o2) {
return o1.getName().compareTo(o2.getName());
}
});
} else {
Arrays.sort(files, new Comparator<File>() {
@Override
public int compare(File o1, File o2) {
return o2.getName().compareTo(o1.getName());
}
});
}
// 输出
printFiles(files, 0);
}
private void printFiles(File[] files, int depth) {
for (File file : files) {
if (file.isDirectory()) {
System.out.println(getIndent(depth) + file.getName() + "/");
printFiles(file.listFiles(), depth + 1);
} else {
System.out.println(getIndent(depth) + file.getName());
}
}
}
private String getIndent(int depth) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < depth; i++) {
sb.append(" ");
}
return sb.toString();
}
}
```
这个实现首先对目录下的文件和文件夹按照类型排序,先文件夹在前,文件在后,然后根据传入的sort参数按名称排序,最后输出整个目录树。其中,使用递归来输出子目录的目录树。
阅读全文
相关推荐
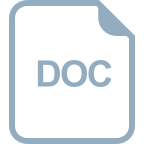
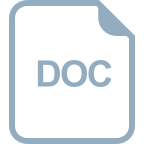
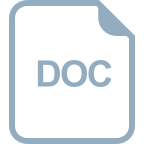
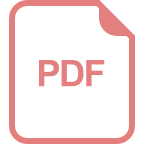
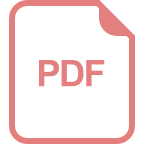
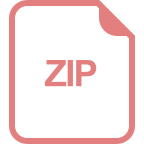
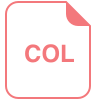





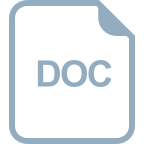
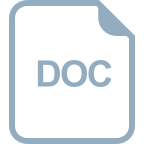
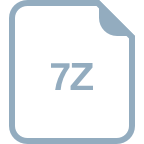
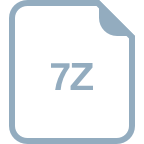
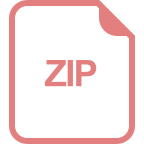
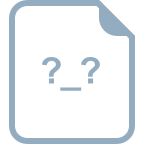