CString添加字符串
时间: 2024-03-31 11:33:57 浏览: 156
要向CString对象中添加字符串,可以使用CString类中的`Append`或`operator+=`函数。这两个函数都可以将一个字符串添加到另一个字符串的末尾。
例如,假设存在一个CString对象str1,其中包含字符串"Hello, ",现在需要将字符串"world!"添加到该对象中,可以使用以下方法:
```
CString str1 = "Hello, ";
CString str2 = "world!";
str1.Append(str2); // 使用Append函数将str2添加到str1的末尾
```
或者,使用`operator+=`函数,将字符串直接添加到str1的末尾:
```
CString str1 = "Hello, ";
CString str2 = "world!";
str1 += str2; // 使用operator+=函数将str2添加到str1的末尾
```
最终,str1的内容将为"Hello, world!"。
相关问题
CString添加字符
在 MFC 中,`CString` 类型是用来处理字符串的,它是 C++ 的类模板,提供了一系列方便的操作字符串的方法。如果你想要在 `CString` 对象中添加字符,可以使用多种方法:
1. **使用 `+=` 运算符**:
```cpp
CString str;
str += 'a'; // 添加单个字符
str += "Hello"; // 添加另一个字符串
```
这将直接在当前字符串的末尾追加新的字符或字符串。
2. **使用 `append` 函数**:
```cpp
CString str;
str.Append('a');
str.Append("Hello");
```
`append` 函数的功能与 `+=` 相似,也是将指定的字符或字符串添加到字符串末尾。
3. **使用 `MakeAlloc` 和 `SetAt`**:
如果需要动态扩展长度,可以先使用 `MakeAlloc` 增加内存容量,然后通过索引来设置特定位置的字符:
```cpp
str.MakeAlloc(50); // 假设初始容量为20
str.SetAt(0, 'a'); // 在第一个位置插入字符
str.SetAt(4, 'b'); // 在第五个位置插入字符
```
这里需要注意的是,`SetAt` 要谨慎使用,因为它可能会改变字符串的实际长度,因此最好配合 `GetLength()` 或其他方式检查有效索引。
cstring字符串的拆分
在C++中,`cstring`库主要用于处理宽字符数组,但它本身并不直接提供字符串拆分的功能。如果你想要在`cstring`上下文中进行字符串拆分,通常需要借助于标准库中的`std::string`或更现代的C++11及以上版本,使用`std::istringstream`或`std::string::find`等方法。
例如,如果你有一个宽字符字符串,并想按照特定字符分割成多个部分,你可以这样做:
```cpp
#include <cwchar>
#include <vector>
#include <sstream>
std::wstring wide_str = L"Hello,World;This,is,C-string";
std::vector<std::wstring> parts;
std::wstringstream ss(wide_str);
std::wstring part;
while (std::getline(ss, part, ';')) {
parts.push_back(part);
}
// parts now contains ["Hello,World", "This,is", "C-string"]
```
在这个例子中,我们创建了一个`std::wstringstream`,然后通过`std::getline`函数每次读取到分隔符`;`时停止,将读取的部分添加到结果向量中。
阅读全文
相关推荐
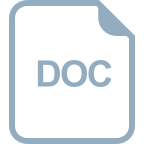
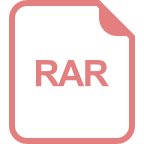
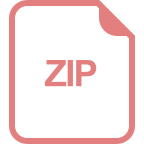
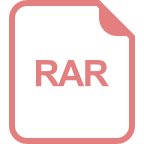
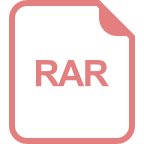
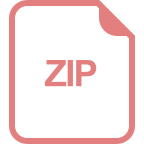
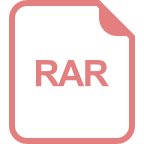
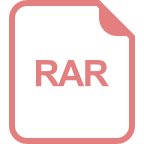
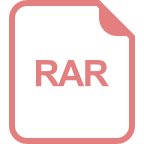
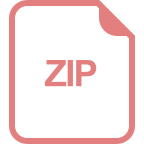
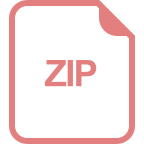
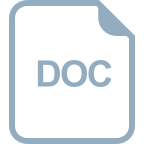


