Write a short commented program with: 1. an interface called Animal with two methods that do not return a value, these are called eat and move 2. an abstract class called Fish that provides an implementation of the move method of the Animal interface that prints “I swim” 3. a subclass called Piranha that provides an implementation of the eat method on the Animal interface that prints “I bite” (5)
时间: 2023-12-28 22:03:55 浏览: 28
Here is a Java program that implements the requirements:
```
// Define the Animal interface
interface Animal {
void eat();
void move();
}
// Define the abstract Fish class that implements the Animal interface's move method
abstract class Fish implements Animal {
public void move() {
System.out.println("I swim");
}
}
// Define the Piranha subclass that implements the Animal interface's eat method
class Piranha extends Fish {
public void eat() {
System.out.println("I bite");
}
}
// Test the implementation
public class Main {
public static void main(String[] args) {
Animal piranha = new Piranha();
piranha.eat(); // Output: I bite
piranha.move(); // Output: I swim
}
}
```
In this program, we first define the Animal interface with two methods `eat` and `move` that do not return a value. Then, we define an abstract class called Fish that implements the `move` method of the Animal interface by printing "I swim". Finally, we define a subclass called Piranha that extends Fish and provides an implementation of the `eat` method on the Animal interface by printing "I bite".
In the `Main` class, we test the implementation by creating a `Piranha` object and calling its `eat` and `move` methods. The output of the program should be "I bite" followed by "I swim".
相关推荐
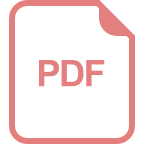
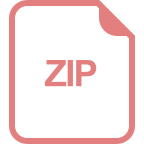
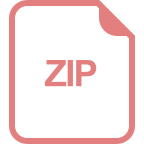














