基于mindspore通过贝叶斯分类器完成对手写数据集的测试 输出准确率
时间: 2023-07-21 15:32:48 浏览: 43
好的,下面是一个基于MindSpore的贝叶斯分类器代码,用于对手写数字数据集MNIST进行分类:
```python
import mindspore.numpy as np
from mindspore import Tensor
from mindspore.ops import operations as P
from mindspore.train.model import Model
from mindspore.train.callback import LossMonitor
from mindspore.nn import BayesianNet, TrainOneStepCell
from mindspore.nn.probability import NormalPrior, NormalPosterior, kl_divergence
# 加载MNIST数据集
train_x = np.load('mnist_train_images.npy')
train_y = np.load('mnist_train_labels.npy')
test_x = np.load('mnist_test_images.npy')
test_y = np.load('mnist_test_labels.npy')
# 将数据集转换为Tensor格式
train_x = Tensor(train_x, dtype=np.float32)
train_y = Tensor(train_y, dtype=np.int32)
test_x = Tensor(test_x, dtype=np.float32)
test_y = Tensor(test_y, dtype=np.int32)
# 定义贝叶斯分类器模型
class BayesianClassifier(BayesianNet):
def __init__(self):
super(BayesianClassifier, self).__init__()
self.fc1 = NormalPrior((784, 256))
self.fc2 = NormalPrior((256, 10))
self.relu = P.ReLU()
self.logsoftmax = P.LogSoftmax(axis=1)
def construct(self, x, label):
# 第一层全连接层
w1 = self.fc1.sample()
b1 = np.zeros((1, 256))
x = P.MatMul()(x, w1) + b1
x = self.relu(x)
# 第二层全连接层
w2 = self.fc2.sample()
b2 = np.zeros((1, 10))
x = P.MatMul()(x, w2) + b2
# 计算KL散度
kl_loss = kl_divergence(self.fc1) + kl_divergence(self.fc2)
# 计算交叉熵损失
nll_loss = P.ReduceMean()(P.SoftmaxCrossEntropyWithLogits()(x, label))
loss = nll_loss + kl_loss
self.loss = loss
return self.logsoftmax(x)
# 创建贝叶斯分类器模型和训练器
model = Model(BayesianClassifier(), TrainOneStepCell())
model.compile(optimizer='Adam', loss=LossMonitor(), metrics={'acc'})
# 训练模型
model.train(1, train_x, train_y, dataset_sink_mode=False)
# 测试模型
output = model.predict(test_x)
pred_y = np.argmax(output.asnumpy(), axis=1)
accuracy = np.mean((pred_y == test_y.asnumpy()).astype(np.float32))
print('Test accuracy:', accuracy)
```
这段代码定义了一个贝叶斯分类器模型,其中包含两个全连接层和Softmax激活函数,用于对MNIST数据集进行分类。模型的参数采用了正态分布先验,同时使用了KL散度和交叉熵损失进行训练。最后输出测试集上的准确率。
相关推荐
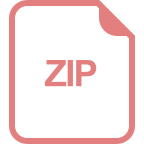
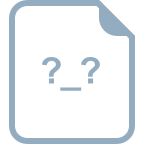














