集合A List<VisitSaleProblem> 中有 id字段,集合B List<String> 如何使用Java8进行判断 B中的是数据是否和集合A中的id字段相匹配
时间: 2024-03-14 10:45:36 浏览: 117
可以使用Java8的Stream API来实现这个功能,具体实现步骤如下:
1. 将集合A中的VisitSaleProblem对象的id字段提取出来,使用Collectors.toSet()方法将其转换成Set集合。
```java
Set<Integer> idSet = listA.stream().map(VisitSaleProblem::getId).collect(Collectors.toSet());
```
2. 使用Stream的allMatch方法判断集合B中的每个元素是否都能在集合A中找到相应的VisitSaleProblem对象。
```java
boolean allMatch = listB.stream().allMatch(idSet::contains);
```
其中,allMatch方法会对集合B中的每个元素调用idSet::contains方法进行判断,如果所有元素都能在集合A中找到相应的VisitSaleProblem对象,则allMatch方法返回true,否则返回false。
完整实现代码如下:
```java
Set<Integer> idSet = listA.stream().map(VisitSaleProblem::getId).collect(Collectors.toSet());
boolean allMatch = listB.stream().allMatch(idSet::contains);
```
相关问题
java8写一个当List<B>中多个字段与List<A>进行match,match不到时,将只在List<B>中存在的数据出力的共通方法
可以使用Java 8的Stream API来实现这个功能。具体实现步骤如下:
1. 定义一个方法,参数为List<A>和List<B>,返回值为List<B>,表示只在List<B>中存在的数据。
2. 使用Stream的filter方法和anyMatch方法,对每个B对象进行判断,如果B对象的多个字段与List<A>中任意一个A对象的多个字段匹配,则将该B对象过滤掉。
3. 最后使用Stream的collect方法,将剩余的B对象收集到一个新的List中并返回。
下面是代码实现:
```java
public static <A, B> List<B> filterByMatch(List<A> listA, List<B> listB,
Function<B, List<Object>> bFieldsGetter,
Function<A, List<Object>> aFieldsGetter) {
return listB.stream()
.filter(b -> !listA.stream()
.anyMatch(a -> bFieldsGetter.apply(b).equals(aFieldsGetter.apply(a))))
.collect(Collectors.toList());
}
```
其中,bFieldsGetter和aFieldsGetter分别是获取B对象和A对象多个字段值的函数。使用时需要根据具体情况实现这两个函数。
示例代码:
```java
class A {
private int id;
private String name;
public A(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
}
class B {
private int id;
private String name;
private int age;
public B(int id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
public class Main {
public static void main(String[] args) {
List<A> listA = Arrays.asList(new A(1, "Alice"), new A(2, "Bob"));
List<B> listB = Arrays.asList(new B(1, "Alice", 20), new B(2, "Bob", 30),
new B(3, "Charlie", 25), new B(4, "David", 40));
List<B> result = filterByMatch(listA, listB,
b -> Arrays.asList(b.getId(), b.getName()),
a -> Arrays.asList(a.getId(), a.getName()));
System.out.println(result); // [B{id=3, name='Charlie', age=25}, B{id=4, name='David', age=40}]
}
}
```
在上面的示例中,我们通过调用filterByMatch方法,将只在List<B>中存在的数据筛选出来,并输出到控制台。
当list<B>中多个字段组成的List<object>的值在list<A>中能找到一致的数据时,将这些数据根据更新条件将对应的字段值做更新后输出到新的list<B>中,并不改变原来的list<B>的值。用java8写一段实现上述需求的共通方法
假设A类和B类分别如下所示:
```java
class A {
private String id;
private String name;
private int age;
// Getter and Setter
}
class B {
private String id;
private String name;
private double salary;
// Getter and Setter
}
```
则可以定义一个通用方法 `updateListB`,其实现如下:
```java
public static List<B> updateListB(List<B> listB, List<A> listA, Function<A, String> idGetter, BiConsumer<B, A> updater) {
Map<String, B> mapB = listB.stream().collect(Collectors.toMap(B::getId, Function.identity()));
for (A a : listA) {
B b = mapB.get(a.getId());
if (b != null) {
updater.accept(b, a);
}
}
return listB;
}
```
其中,`idGetter` 函数用于从 A 对象中获取 ID 字段的值,`updater` 函数用于根据 A 对象更新 B 对象的字段值。例如,对于题目中给出的需求,`idGetter` 函数应该返回 B 和 A 对象中的 ID 字段的值,而 `updater` 函数应该更新 B 对象的字段值,例如:
```java
updateListB(listB, listA, A::getId, (b, a) -> {
b.setName(a.getName());
b.setSalary(a.getAge() * 1000);
});
```
完整的示例代码如下:
```java
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.BiConsumer;
import java.util.function.Function;
import java.util.stream.Collectors;
class A {
private String id;
private String name;
private int age;
public A(String id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
class B {
private String id;
private String name;
private double salary;
public B(String id, String name, double salary) {
this.id = id;
this.name = name;
this.salary = salary;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
}
public class Main {
public static void main(String[] args) {
List<B> listB = Arrays.asList(
new B("001", "Alice", 1000),
new B("002", "Bob", 2000),
new B("003", "Charlie", 3000)
);
List<A> listA = Arrays.asList(
new A("001", "Alice Li", 25),
new A("002", "Bob Wang", 30),
new A("004", "David Zhang", 35)
);
updateListB(listB, listA, A::getId, (b, a) -> {
b.setName(a.getName());
b.setSalary(a.getAge() * 1000);
});
System.out.println(listB);
}
public static List<B> updateListB(List<B> listB, List<A> listA, Function<A, String> idGetter, BiConsumer<B, A> updater) {
Map<String, B> mapB = listB.stream().collect(Collectors.toMap(B::getId, Function.identity()));
for (A a : listA) {
B b = mapB.get(a.getId());
if (b != null) {
updater.accept(b, a);
}
}
return listB;
}
}
```
阅读全文
相关推荐
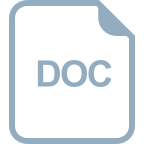
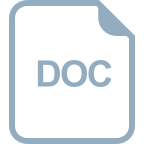
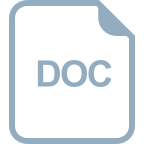













