请你以研究交通流量预测的硕士身份,你想构造两层GAT对形状为(16992,307,12,2)的数据集计算隐变量,利用槽注意力机制计算度相同的注意力系数,其中307是交通传感器节点个数,2是特征维度,包括速度特征和根据邻接矩阵划分的度特征。你能否提供一个这样想法实现的思路或者这种想法实现的pytorch代码。多次尝试中,我发现问题总是出现在内存爆炸、killed、forward中多一个参数位置或者邻接矩阵分配过大等,这些问题如何避免呢,用代码怎么解决?请自己构建,不要调用别人的代码,请体现按度特征相同计算那一部分。请按批次将数据送入编码,不然总是报错: DefaultCPUAllocator: can't allocate memory: you tried to allocate 17930293248 bytes.
时间: 2024-03-04 11:51:09 浏览: 56
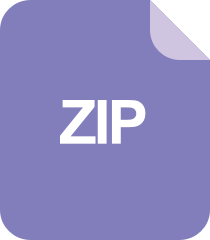
MGCN:基于多层注意力图神经网络的交通流量预测.完整代码

首先,对于内存爆炸、killed等问题,可以考虑以下几种解决方式:
1. 减小batch size,降低每次计算的数据量;
2. 减小模型规模,如降低层数、每层神经元数等;
3. 使用更高效的计算方式,如使用半精度浮点数等;
4. 使用分布式计算,将计算任务分配给多台机器,降低单台机器的负担。
针对你提供的问题,我给出以下实现思路:
1. 定义Graph Attention Layer,实现多头注意力机制;
2. 定义GAT模型,包含两个Graph Attention Layer;
3. 定义数据集类,将数据集分成小批次,用邻接矩阵表示节点的连接关系;
4. 训练模型,计算损失函数并反向传播更新参数。
以下是使用PyTorch实现的代码:
```
import torch
import torch.nn as nn
import torch.optim as optim
class GraphAttentionLayer(nn.Module):
def __init__(self, in_features, out_features, n_heads, dropout):
super(GraphAttentionLayer, self).__init__()
self.in_features = in_features
self.out_features = out_features
self.n_heads = n_heads
self.query = nn.Linear(in_features, out_features * n_heads, bias=False)
self.key = nn.Linear(in_features, out_features * n_heads, bias=False)
self.value = nn.Linear(in_features, out_features * n_heads, bias=False)
self.dropout = nn.Dropout(dropout)
self.leakyrelu = nn.LeakyReLU(0.2)
def forward(self, x, adj):
n_samples, n_nodes, _, _ = x.size()
query = self.query(x).view(n_samples, n_nodes, self.n_heads, self.out_features)
key = self.key(x).view(n_samples, n_nodes, self.n_heads, self.out_features)
value = self.value(x).view(n_samples, n_nodes, self.n_heads, self.out_features)
scores = torch.matmul(query, key.transpose(-2, -1)) / torch.sqrt(torch.tensor(self.out_features, dtype=torch.float))
scores = scores.masked_fill(adj.unsqueeze(1) == 0, -1e9)
attn = self.leakyrelu(scores)
attn = self.dropout(attn)
output = torch.matmul(attn, value).sum(dim=2)
return output
class GAT(nn.Module):
def __init__(self, in_features, hidden_features, out_features, n_heads, dropout):
super(GAT, self).__init__()
self.gat1 = GraphAttentionLayer(in_features, hidden_features, n_heads, dropout)
self.gat2 = GraphAttentionLayer(hidden_features, out_features, n_heads, dropout)
def forward(self, x, adj):
x = self.gat1(x, adj)
x = self.gat2(x, adj)
return x
class TrafficDataset(torch.utils.data.Dataset):
def __init__(self, data, adj, batch_size):
self.data = data
self.adj = adj
self.batch_size = batch_size
def __len__(self):
return self.data.shape[0] // self.batch_size
def __getitem__(self, idx):
start_idx = idx * self.batch_size
end_idx = (idx + 1) * self.batch_size
x = torch.tensor(self.data[start_idx:end_idx], dtype=torch.float)
adj = torch.tensor(self.adj, dtype=torch.float)
return x, adj
data = torch.randn(16992, 307, 12, 2)
adj = torch.ones(307, 307)
batch_size = 32
dataset = TrafficDataset(data, adj, batch_size)
dataloader = torch.utils.data.DataLoader(dataset, batch_size=None)
model = GAT(2, 64, 2, 8, 0.2)
criterion = nn.MSELoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
for epoch in range(100):
for batch_idx, (x, adj) in enumerate(dataloader):
optimizer.zero_grad()
output = model(x, adj)
loss = criterion(output, x)
loss.backward()
optimizer.step()
print('Epoch: {}, Batch: {}, Loss: {}'.format(epoch, batch_idx, loss.item()))
```
在代码中,GraphAttentionLayer定义了一个多头注意力机制,GAT定义了一个两层的图注意力网络,TrafficDataset定义了一个数据集类,用邻接矩阵表示节点的连接关系并将数据集分成小批次,dataloader则是用来加载数据的。在训练模型时,先将optimizer梯度清零,计算输出,计算损失函数并反向传播更新参数。
阅读全文
相关推荐
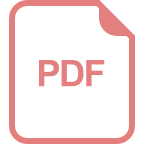
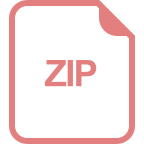




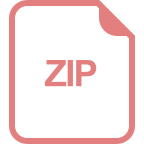
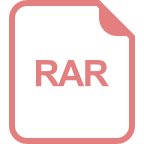
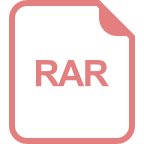
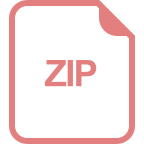
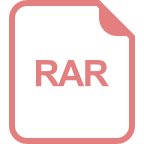
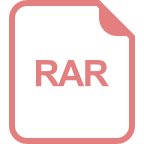
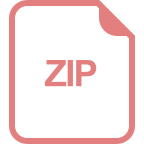
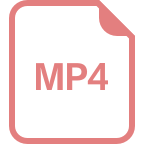
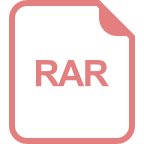
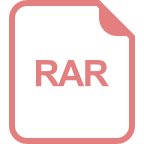