python不调用库实现留出法验证ID3决策树,以titanic数据集为例
时间: 2024-01-28 11:03:17 浏览: 89
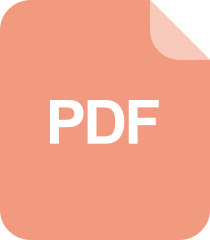
python数据分析之决策树实践
由于留出法验证是一种常见的机器学习验证方法,因此在Python中实现留出法验证ID3决策树也是很常见的。在这里,我们将使用titanic数据集作为例子来演示如何使用Python实现留出法验证ID3决策树。
步骤如下:
1. 导入数据集并进行预处理
首先,我们需要导入titanic数据集,并对其进行预处理,以便将其用于ID3决策树的训练和验证。我们可以使用Pandas库来导入和处理数据集。具体步骤如下:
```python
import pandas as pd
# 导入数据集
data = pd.read_csv('titanic.csv')
# 删除无用的列
data.drop(['PassengerId', 'Name', 'Ticket', 'Cabin'], axis=1, inplace=True)
# 处理缺失值
data['Age'].fillna(data['Age'].median(), inplace=True)
data['Embarked'].fillna(data['Embarked'].mode()[0], inplace=True)
# 将类别型变量转换为数值型变量
data['Sex'].replace(['male', 'female'], [0, 1], inplace=True)
data['Embarked'].replace(['S', 'C', 'Q'], [0, 1, 2], inplace=True)
# 将数据集拆分为训练集和测试集
train_data = data[:800]
test_data = data[800:]
```
在这里,我们使用了Pandas库中的read_csv函数来导入titanic数据集。然后,我们删除了无用的列,并对缺失值进行了处理。接下来,我们将类别型变量转换为数值型变量,并将数据集拆分为训练集和测试集。
2. 定义ID3决策树算法
接下来,我们需要定义ID3决策树算法,以便对训练集进行训练,并生成一个决策树模型。具体实现如下:
```python
import math
def calculate_entropy(data):
"""
计算数据集的熵
"""
n = len(data)
count = data['Survived'].value_counts()
p1 = count[1] / n
p0 = count[0] / n
if p1 == 0 or p0 == 0:
return 0
else:
return - (p1 * math.log2(p1) + p0 * math.log2(p0))
def calculate_conditional_entropy(data, feature):
"""
计算数据集关于某个特征的条件熵
"""
n = len(data)
values = data[feature].unique()
entropy = 0
for v in values:
subset = data[data[feature] == v]
entropy += len(subset) / n * calculate_entropy(subset)
return entropy
def choose_best_feature(data, features):
"""
选择最优特征
"""
n = len(data)
base_entropy = calculate_entropy(data)
best_info_gain = 0
best_feature = None
for feature in features:
entropy = calculate_conditional_entropy(data, feature)
info_gain = base_entropy - entropy
if info_gain > best_info_gain:
best_info_gain = info_gain
best_feature = feature
return best_feature
def create_decision_tree(data, features):
"""
创建决策树
"""
count = data['Survived'].value_counts()
if len(count) == 1:
return count.index[0]
if len(features) == 0:
return count.idxmax()
best_feature = choose_best_feature(data, features)
tree = {best_feature: {}}
values = data[best_feature].unique()
for v in values:
subset = data[data[best_feature] == v]
subtree = create_decision_tree(subset, features - {best_feature})
tree[best_feature][v] = subtree
return tree
```
在这里,我们定义了四个函数来实现ID3决策树算法。具体来说:
- calculate_entropy函数用于计算数据集的熵;
- calculate_conditional_entropy函数用于计算数据集关于某个特征的条件熵;
- choose_best_feature函数用于选择最优特征;
- create_decision_tree函数用于创建决策树。
3. 实现留出法验证
最后,我们需要实现留出法验证来评估ID3决策树的性能。具体实现如下:
```python
def predict(tree, data):
"""
预测
"""
if type(tree) != dict:
return tree
feature = list(tree.keys())[0]
value = data[feature]
subtree = tree[feature][value]
return predict(subtree, data)
def evaluate(tree, test_data):
"""
评估
"""
n = len(test_data)
correct = 0
for i in range(n):
data = test_data.iloc[i]
label = predict(tree, data)
if label == data['Survived']:
correct += 1
return correct / n
# 训练
features = set(train_data.columns) - {'Survived'}
tree = create_decision_tree(train_data, features)
# 验证
accuracy = evaluate(tree, test_data)
print('Accuracy:', accuracy)
```
在这里,我们首先定义了predict函数来预测测试数据集中的标签。然后,我们定义了evaluate函数来评估决策树模型的性能。最后,我们使用训练集训练了一个决策树模型,并在测试集上进行了验证,并输出了模型的准确率。
总结
在本文中,我们演示了如何使用Python实现留出法验证ID3决策树,并以titanic数据集为例进行了演示。这是一个非常基础的例子,但它可以帮助您了解如何使用Python实现留出法验证ID3决策树。如果您需要进一步了解如何使用Python实现其他机器学习算法,请参考其他相关文章。
阅读全文
相关推荐
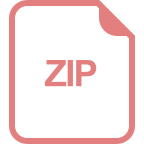
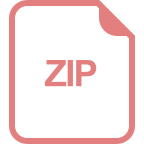















