如何在Python中生成随机颜色?
时间: 2024-04-25 12:18:41 浏览: 427
在Python中生成随机颜色有多种方法,以下是两种常用的方法:
1. 使用random模块生成RGB随机颜色[^1]:
```python
import random
# 生成随机RGB颜色
r = random.randint(0, 255)
g = random.randint(0, 255)
b = random.randint(0, 255)
# 将RGB值转换为十六进制颜色表示
color = '#{:02x}{:02x}{:02x}'.format(r, g, b)
print("随机颜色:", color)
```
2. 使用matplotlib库生成随机颜色[^2]:
```python
import matplotlib.pyplot as plt
import random
# 生成随机颜色
color = random.choice(list(plt.cm.colors.CSS4_COLORS.keys()))
print("随机颜色:", color)
```
这两种方法都可以生成随机的颜色值,你可以根据自己的需求选择其中一种方法来使用。
相关问题
如何在Python中生成随机背景颜色?
在Python中,我们可以利用PIL(Python Imaging Library)库来生成随机背景颜色。这里是一个简单的例子,它创建了一个RGB颜色模式下的随机颜色:
```python
from PIL import ImageDraw
def generate_random_color():
# 从0到255范围内随机选择三个通道的值
r = random.randint(0, 255)
g = random.randint(0, 255)
b = random.randint(0, 255)
return (r, g, b)
# 创建一个黑色的Image对象,并添加一个draw对象
image = Image.new('RGB', (500, 500), (0, 0, 0))
draw = ImageDraw.Draw(image)
# 使用生成的随机颜色填充整个画布
color = generate_random_color()
draw.rectangle((0, 0, image.width, image.height), fill=color)
# 显示生成的图像
image.show()
# 如果需要保存图片,可以使用save方法
image.save("random_background.png")
```
这个脚本会在一个500x500像素的图片上生成一个随机背景。如果你想改变背景颜色的生成规则,只需调整`generate_random_color()`函数即可。
python生成随机颜色
在Python中,可以使用random模块来生成随机颜色。下面是一个简单的示例代码:
```python
import random
def generate_random_color():
r = random.randint(0, 255)
g = random.randint(0, 255)
b = random.randint(0, 255)
return (r, g, b)
color = generate_random_color()
print("随机生成的颜色为:", color)
```
这段代码使用random模块的randint函数来生成0到255之间的随机整数作为RGB颜色的取值范围。然后将这三个随机整数作为元组返回,即表示一个随机颜色。你可以根据需要将这个颜色值用于你的项目中。
阅读全文
相关推荐
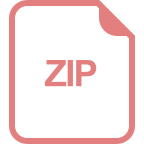
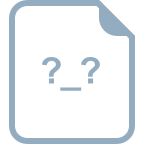












