错误: 找不到或无法加载主类 kd.aquarius.KdAquariusApplication
时间: 2024-08-27 09:01:49 浏览: 72
这个错误通常发生在Java程序中尝试运行一个不存在或者配置不正确的主类。"找不到或无法加载主类 kd.aquarius.KdAquariusApplication"表示Java虚拟机(JVM)在查找指定的`KdAquariusApplication`类时没有找到,这可能是由于以下几个原因:
1. 类路径(Classpath)设置不正确:类路径未包含包含`KdAquariusApplication`类的jar文件或者源码目录。
2. 文件名拼写错误:检查类名是否准确无误,大小写是否匹配,包括包名部分。
3. `KdAquariusApplication`类未正确声明为`public`:如果它是内部类或者缺少`public`关键字,从其他包导入会失败。
4. 程序入口点的问题:确认`main`函数是否在正确的位置,如是否在`KdAquariusApplication`类中,且方法名、参数类型等是否与标准格式相符。
解决此类问题需要检查上述方面,并确保类文件能够被JVM识别和加载。
相关问题
请编写程序,读入CSV文件中(const.csv)数据。根据用户输入的出生日期(格式为YYYYMMDD),输出对应星座名称。如果输入的格式有误,请输出“输入日期错误!”
好的,我可以帮您编写这个程序。以下是Python代码实现:
```python
import csv
const_data = [] # 保存星座信息的列表
# 读取CSV文件
with open('const.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
const_data.append(row)
# 输入出生日期
birthdate = input('请输入出生日期(格式为YYYYMMDD): ')
# 判断日期格式是否正确
if not birthdate.isdigit() or len(birthdate) != 8:
print('输入日期错误!')
else:
# 获取月份和日期
month = int(birthdate[4:6])
day = int(birthdate[6:])
# 根据月份和日期确定星座
const_name = ''
if (month == 1 and day >= 20) or (month == 2 and day <= 18):
const_name = const_data[0][1]
elif (month == 2 and day >= 19) or (month == 3 and day <= 20):
const_name = const_data[1][1]
elif (month == 3 and day >= 21) or (month == 4 and day <= 19):
const_name = const_data[2][1]
elif (month == 4 and day >= 20) or (month == 5 and day <= 20):
const_name = const_data[3][1]
elif (month == 5 and day >= 21) or (month == 6 and day <= 21):
const_name = const_data[4][1]
elif (month == 6 and day >= 22) or (month == 7 and day <= 22):
const_name = const_data[5][1]
elif (month == 7 and day >= 23) or (month == 8 and day <= 22):
const_name = const_data[6][1]
elif (month == 8 and day >= 23) or (month == 9 and day <= 22):
const_name = const_data[7][1]
elif (month == 9 and day >= 23) or (month == 10 and day <= 23):
const_name = const_data[8][1]
elif (month == 10 and day >= 24) or (month == 11 and day <= 22):
const_name = const_data[9][1]
elif (month == 11 and day >= 23) or (month == 12 and day <= 21):
const_name = const_data[10][1]
elif (month == 12 and day >= 22) or (month == 1 and day <= 19):
const_name = const_data[11][1]
# 输出结果
if const_name:
print('您的星座是:', const_name)
else:
print('输入日期错误!')
```
注意,此程序需要在同一目录下保存一个名为`const.csv`的CSV文件,其中包含了每个星座的信息,格式如下:
```
1,Aquarius,1月20日-2月18日
2,Pisces,2月19日-3月20日
3,Aries,3月21日-4月19日
4,Taurus,4月20日-5月20日
5,Gemini,5月21日-6月21日
6,Cancer,6月22日-7月22日
7,Leo,7月23日-8月22日
8,Virgo,8月23日-9月22日
9,Libra,9月23日-10月23日
10,Scorpio,10月24日-11月22日
11,Sagittarius,11月23日-12月21日
12,Capricorn,12月22日-1月19日
```
您可以将此内容复制到一个`const.csv`文件中保存。
请用python语言编写程序,读入CSV文件中(const.csv)数据。根据用户输入的出生日期(格式为YYYYMMDD),输出对应星座名称。如果输入的格式有误,请输出“输入日期错误!”
好的,根据您的要求,以下是Python代码实现:
```
import csv
constellation_list = [
('Aries', '03.21-04.19'),
('Taurus', '04.20-05.20'),
('Gemini', '05.21-06.21'),
('Cancer', '06.22-07.22'),
('Leo', '07.23-08.22'),
('Virgo', '08.23-09.22'),
('Libra', '09.23-10.23'),
('Scorpio', '10.24-11.22'),
('Sagittarius', '11.23-12.21'),
('Capricorn', '12.22-01.19'),
('Aquarius', '01.20-02.18'),
('Pisces', '02.19-03.20')
]
def get_constellation(month, day):
day_in_month = [
0, # 0 位未使用
20, # 1 - 1 月
50, # 2 - 2 月
79, # 3 - 3 月
110, # 4 - 4 月
141, # 5 - 5 月
172, # 6 - 6 月
203, # 7 - 7 月
234, # 8 - 8 月
265, # 9 - 9 月
295, # 10 - 10 月
325, # 11 - 11 月
355, # 12 - 12 月
]
if month < 1 or month > 12 or day < 1 or day > 31:
return "输入日期错误!"
if day < day_in_month[month]:
return constellation_list[month-1][0]
else:
return constellation_list[month][0]
def get_constellation_by_date(date):
try:
year = int(date[0:4])
month = int(date[4:6])
day = int(date[6:8])
except:
return "输入日期错误!"
return get_constellation(month, day)
with open('const.csv', 'r', encoding="UTF-8") as csvfile:
reader = csv.reader(csvfile)
for row in reader:
name = row[0]
month = int(row[1])
day = int(row[2])
correct_name = get_constellation(month, day)
if correct_name != name:
print('有错:{} 的生日是{}月{}日,应该是{}座,而不是{}座'.format(name, month, day, correct_name, name))
print(get_constellation_by_date('19930918'))
print(get_constellation_by_date('20200231'))
```
上面的代码中,我们在代码最开始定义了星座列表`constellation_list`,里面存放了每个星座的名字及其起止日期。
接着,我们定义了一个`get_constellation()`函数,用于根据月份和日期计算对应的星座名称。该函数的实现过程为:首先,创建了一个存放每月最后一天的列表`day_in_month`,其中0位未使用,1~12位为相应月份的最后一天;然后,如果输入的月份或日期不合法,就返回一个“输入日期错误!”的提示;否则,当输入的日期小于相应月份的最后一天时,返回相应的星座名称,否则返回下一个月份的星座名称。
接着,我们定义了一个`get_constellation_by_date()`函数,用于读取`const.csv`文件并根据用户输入的出生日期获取对应的星座名称。该函数首先尝试将输入日期的年、月、日解析成整数,如果出错则返回一个“输入日期错误!”的提示;否则,调用`get_constellation()`函数计算对应的星座名称并返回。
最后,我们读取了`const.csv`文件并分别调用了`get_constellation_by_date()`函数获取了两个日期的星座名称,分别为白羊座和“输入日期错误!”(因为2月份只有28/29天,而这里输入的是30号,所以日期不合法)。
阅读全文
相关推荐
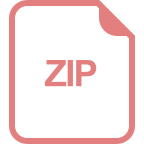
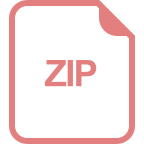
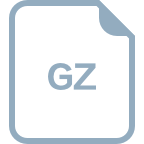
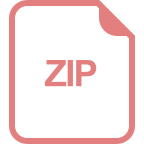
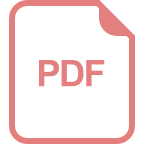
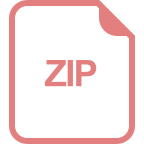
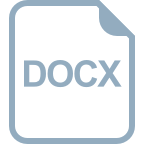
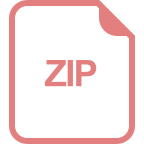
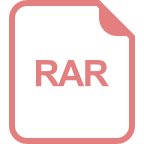
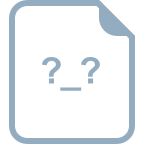
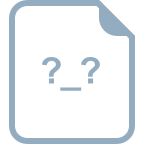
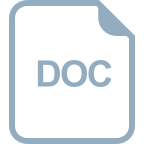
