HT93LC46 读写测试 C51例程
时间: 2023-09-05 22:07:30 浏览: 71
以下是一个简单的C51例程,用于测试HT93LC46芯片的读写功能:
```c
#include <reg51.h>
#define SDA P1_1 // HT93LC46串行数据线
#define SCL P1_0 // HT93LC46串行时钟线
// HT93LC46芯片命令定义
#define HT93LC46_CMD_READ 0b00000011 // 读取数据命令
#define HT93LC46_CMD_WRITE 0b00000010 // 写入数据命令
// 函数:启动I2C总线
void i2c_start() {
SDA = 1;
SCL = 1;
SDA = 0;
SCL = 0;
}
// 函数:停止I2C总线
void i2c_stop() {
SDA = 0;
SCL = 1;
SDA = 1;
}
// 函数:发送一个字节的数据到HT93LC46
void i2c_sendByte(unsigned char data) {
unsigned char i;
for (i = 0; i < 8; i++) {
SDA = (data & (0x80 >> i)) ? 1 : 0;
SCL = 1;
SCL = 0;
}
// 等待HT93LC46应答
SDA = 1;
SCL = 1;
while (SDA == 1);
SCL = 0;
}
// 函数:从HT93LC46读取一个字节的数据
unsigned char i2c_receiveByte() {
unsigned char i, data = 0;
for (i = 0; i < 8; i++) {
SCL = 1;
data |= (SDA ? (0x80 >> i) : 0);
SCL = 0;
}
return data;
}
// 函数:向HT93LC46写入一个字节的数据
void ht93lc46_writeByte(unsigned char address, unsigned char data) {
i2c_start();
i2c_sendByte(HT93LC46_CMD_WRITE);
i2c_sendByte(address);
i2c_sendByte(data);
i2c_stop();
}
// 函数:从HT93LC46读取一个字节的数据
unsigned char ht93lc46_readByte(unsigned char address) {
unsigned char data;
i2c_start();
i2c_sendByte(HT93LC46_CMD_READ);
i2c_sendByte(address);
i2c_start();
data = i2c_receiveByte();
i2c_stop();
return data;
}
void main() {
unsigned char address = 0x00; // HT93LC46地址
unsigned char writeData = 0x55; // 写入的数据
unsigned char readData;
// 向HT93LC46写入数据
ht93lc46_writeByte(address, writeData);
// 从HT93LC46读取数据
readData = ht93lc46_readByte(address);
// 检查读取的数据是否与写入的数据一致
if (readData == writeData) {
// 读写测试通过
// 在此处可以添加其他操作或逻辑
} else {
// 读写测试失败
// 在此处可以添加错误处理代码
}
while (1) {
// 主循环
}
}
```
这个例程使用了C51单片机的GPIO口来模拟HT93LC46的串行数据线(SDA)和串行时钟线(SCL)。通过i2c_start()、i2c_stop()、i2c_sendByte()和i2c_receiveByte()等函数来实现I2C通信协议。
在main()函数中,首先使用ht93lc46_writeByte()函数向HT93LC46芯片的指定地址写入一个字节的数据(writeData)。然后使用ht93lc46_readByte()函数从相同的地址读取一个字节的数据,并将其保存在readData变量中。最后,通过比较读取的数据与写入的数据,判断读写测试是否通过。
请注意,以上只是一个简单的例程,实际应用中可能需要根据具体情况进行适当的修改和扩展。另外,确保正确连接硬件并正确配置C51单片机的引脚和时钟设置。
相关推荐
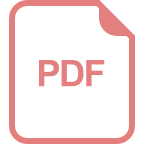
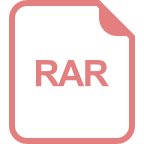














