这段代码有什么问题 <table border = 1> <tr> <th>编号</th> <th>姓名</th> <th>性别</th> <th>年龄</th> </tr> <?php $con = mysql_connect("localhost",'root','123456'); //数据库服务器 mysql_select_db("spark",$con); //找到数据库 $res = mysql_query("select * from student",$con); //写sql语句
时间: 2023-11-16 12:47:39 浏览: 69
这段代码存在几个问题:
1. 使用了已经过时的 MySQL 函数:`mysql_connect`、`mysql_select_db`、`mysql_query`。建议使用现代的数据库连接方式,如 PDO 或者 MySQLi。
2. 没有对数据库连接错误进行处理。在连接数据库时,应该检查连接是否成功,并在连接失败时进行适当的错误处理。
3. 直接将用户输入的值用于 SQL 查询,存在 SQL 注入的风险。应该使用参数化查询或者预处理语句来处理用户输入,以防止 SQL 注入攻击。
4. 代码没有进行错误处理和异常处理。应该在执行数据库操作时,对可能出现的错误进行适当的处理,如捕获异常并进行错误提示。
5. HTML 表格标签 `<table>` 没有正确关闭,应该在最后加上 `</table>`。
这些问题可能会导致代码的安全性、可靠性和可维护性方面的问题,建议对代码进行修复和改进。
相关问题
用户修改后, 点击相同按钮数据重新渲染 <template> <div id="app"> <div> <span>姓名:</span> <input type="text" /> </div> <div> <span>年龄:</span> <input type="number" /> </div> <div> <span>性别:</span> <select > <option value="男">男</option> <option value="女">女</option> </select> </div> <div> <button >添加/修改</button> </div> <div> <table border="1" cellpadding="10" cellspacing="0" > <tr> <th>序号</th> <th>姓名</th> <th>年龄</th> <th>性别</th> <th>操作</th> </tr> <tr > <td></td> <td></td> <td></td> <td></td> <td> <button >删除</button> <button >编辑</button> </td> </tr> </table> </div> </div> </template> <script> export default { } </script>
你需要在 `<input>` 和 `<select>` 标签上添加 `v-model` 指令来实现双向绑定,以便在用户输入数据时,自动更新数据模型。同时,你需要添加一个数组用于存储用户数据,在点击“添加/修改”按钮时,将数据添加到数组中。还需要在表格中用 `v-for` 指令遍历数组,渲染每个用户的数据,并添加“删除”和“编辑”按钮的功能。具体代码如下:
<template>
<div id="app">
<div>
<span>姓名:</span>
<input type="text" v-model="name" />
</div>
<div>
<span>年龄:</span>
<input type="number" v-model="age" />
</div>
<div>
<span>性别:</span>
<select v-model="gender">
<option value="男">男</option>
<option value="女">女</option>
</select>
</div>
<div>
<button @click="addOrUpdateUser">添加/修改</button>
</div>
<div>
<table border="1" cellpadding="10" cellspacing="0">
<tr>
<th>序号</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
<th>操作</th>
</tr>
<tr v-for="(user, index) in userList" :key="index">
<td>{{ index + 1 }}</td>
<td>{{ user.name }}</td>
<td>{{ user.age }}</td>
<td>{{ user.gender }}</td>
<td>
<button @click="deleteUser(index)">删除</button>
<button @click="editUser(index)">编辑</button>
</td>
</tr>
</table>
</div>
</div>
</template>
<script>
export default {
data() {
return {
name: '',
age: '',
gender: '男',
userList: []
}
},
methods: {
addOrUpdateUser() {
// 新建一个用户对象
const user = {
name: this.name,
age: this.age,
gender: this.gender
}
// 判断是否是修改操作
if (this.editIndex !== undefined) {
// 如果是修改操作,则替换原有用户数据
this.userList.splice(this.editIndex, 1, user)
this.editIndex = undefined
} else {
// 如果是添加操作,则将用户数据添加到数组中
this.userList.push(user)
}
// 清空输入框中的数据
this.name = ''
this.age = ''
this.gender = '男'
},
deleteUser(index) {
// 删除指定位置的用户数据
this.userList.splice(index, 1)
},
editUser(index) {
// 将要修改的用户数据填入输入框
const user = this.userList[index]
this.name = user.name
this.age = user.age
this.gender = user.gender
// 记录当前的编辑位置
this.editIndex = index
}
}
}
</script>
● 点击编辑功能, 把值赋予到输入框上 ● : 用户修改后, 点击相同按钮 <template> <div id="app"> <div> <span>姓名:</span> <input type="text" /> </div> <div> <span>年龄:</span> <input type="number" /> </div> <div> <span>性别:</span> <select > <option value="男">男</option> <option value="女">女</option> </select> </div> <div> <button >添加/修改</button> </div> <div> <table border="1" cellpadding="10" cellspacing="0" > <tr> <th>序号</th> <th>姓名</th> <th>年龄</th> <th>性别</th> <th>操作</th> </tr> <tr > <td></td> <td></td> <td></td> <td></td> <td> <button >删除</button> <button >编辑</button> </td> </tr> </table> </div> </div> </template> <script> export default { } </script>
可以使用 Vue.js 来实现这个功能,具体的代码如下:
<template>
<div id="app">
<div>
<span>姓名:</span>
<input type="text" v-model="name" />
</div>
<div>
<span>年龄:</span>
<input type="number" v-model="age" />
</div>
<div>
<span>性别:</span>
<select v-model="gender">
<option value="男">男</option>
<option value="女">女</option>
</select>
</div>
<div>
<button @click="addOrUpdate">添加/修改</button>
</div>
<div>
<table border="1" cellpadding="10" cellspacing="0">
<tr>
<th>序号</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
<th>操作</th>
</tr>
<tr v-for="(item, index) in dataList" :key="index">
<td>{{ index + 1 }}</td>
<td>{{ item.name }}</td>
<td>{{ item.age }}</td>
<td>{{ item.gender }}</td>
<td>
<button @click="deleteItem(index)">删除</button>
<button @click="editItem(index)">编辑</button>
</td>
</tr>
</table>
</div>
</div>
</template>
<script>
export default {
data() {
return {
name: "",
age: "",
gender: "男",
dataList: [],
editIndex: -1, // 当前正在编辑的数据的索引
};
},
methods: {
addOrUpdate() {
if (this.editIndex === -1) {
// 添加数据
this.dataList.push({
name: this.name,
age: this.age,
gender: this.gender,
});
} else {
// 修改数据
this.dataList[this.editIndex].name = this.name;
this.dataList[this.editIndex].age = this.age;
this.dataList[this.editIndex].gender = this.gender;
this.editIndex = -1;
}
// 清空表单
this.name = "";
this.age = "";
this.gender = "男";
},
deleteItem(index) {
this.dataList.splice(index, 1);
},
editItem(index) {
this.editIndex = index;
// 把当前正在编辑的数据的值赋给表单
this.name = this.dataList[index].name;
this.age = this.dataList[index].age;
this.gender = this.dataList[index].gender;
},
},
};
</script>
在这个代码中,我们使用了 Vue.js 的数据绑定和列表渲染等功能,实现了添加、修改、删除和编辑数据的功能。其中,添加和修改数据的方法是通过判断 editIndex 是否为 -1 来实现的,如果是 -1,就表示当前是添加数据,否则就是修改数据。编辑数据的方法则是把当前正在编辑的数据的值赋给表单,并保存正在编辑的数据的索引。删除数据的方法使用了 splice 方法,根据索引删除指定的数据。
阅读全文
相关推荐
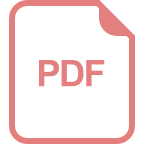
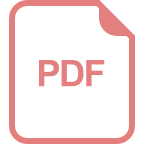
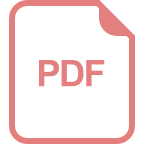



<body> <form action="QueryServlet" method="post"> 请输入用户名:<input type="text" name="userName"/> <input type="submit" value="查询"/> 显示全部 </form> 用户名 密码 积分 注册时间 <c:forEach items="${users }" var="item"> ${item.userName } ${item.password } ${item.integral } ${item.registerTime } 删除 </c:forEach> </body>tomcat 删除一条记录怎么写









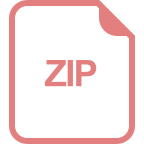