使用stmL432kc芯片,发射IR333C-A 接收PT334-6B ,LCD1602显示屏还有mbed做一个简易的红外脉搏检测系统,请给出代码
时间: 2024-02-25 19:51:15 浏览: 35
以下是一个基于mbed的简易红外脉搏检测系统的代码示例,其中包括了IR发送、接收、LCD显示以及脉搏检测的功能。需要注意的是,由于涉及到硬件连接,代码仅供参考,具体实现还需要根据实际情况进行调整。
```cpp
#include "mbed.h"
#include "TextLCD.h"
#define IR_SEND_PIN PA_0
#define IR_RECEIVE_PIN PA_1
#define LCD_RS_PIN PB_5
#define LCD_E_PIN PB_4
#define LCD_D4_PIN PB_3
#define LCD_D5_PIN PB_2
#define LCD_D6_PIN PA_10
#define LCD_D7_PIN PA_9
#define IR_COMMAND 0x12 // 发送的红外命令
#define IR_ADDRESS 0x34 // 发送的红外地址
DigitalOut irSend(IR_SEND_PIN);
DigitalIn irReceive(IR_RECEIVE_PIN);
TextLCD lcd(LCD_RS_PIN, LCD_E_PIN, LCD_D4_PIN, LCD_D5_PIN, LCD_D6_PIN, LCD_D7_PIN);
Ticker ticker;
volatile int pulseCount = 0;
// 当发现一个下降沿时,统计一个脉搏
void pulseCountCallback() {
pulseCount++;
}
// 发送红外命令
void irSendCommand(uint8_t command) {
irSend = 1;
wait_us(800);
for (int i = 0; i < 8; i++) {
if (command & 0x01) {
irSend = 0;
wait_us(560);
irSend = 1;
wait_us(1690);
} else {
irSend = 0;
wait_us(560);
irSend = 1;
wait_us(560);
}
command >>= 1;
}
irSend = 0;
wait_us(560);
}
// 接收红外命令
uint8_t irReceiveCommand() {
uint8_t command = 0;
uint8_t bitCount = 0;
uint32_t lastTime = 0;
while (1) {
if (irReceive == 0 && lastTime > 0) {
uint32_t pulseWidth = us_ticker_read() - lastTime;
if (pulseWidth > 8000) {
break;
} else if (pulseWidth > 3000) {
bitCount = 0;
} else {
command >>= 1;
if (pulseWidth > 1000) {
command |= 0x80;
}
bitCount++;
if (bitCount >= 8) {
break;
}
}
lastTime = 0;
} else if (irReceive == 1 && lastTime == 0) {
lastTime = us_ticker_read();
}
}
return command;
}
// 每隔1秒钟显示当前的脉搏数
void displayPulseCount() {
lcd.locate(0, 1);
lcd.printf("Pulse: %d", pulseCount);
pulseCount = 0;
}
int main() {
lcd.cls();
lcd.printf("IR Test");
wait(1);
while (1) {
// 发送红外命令
irSendCommand(IR_COMMAND);
// 接收红外命令
uint8_t command = irReceiveCommand();
if (command == IR_COMMAND) {
lcd.cls();
lcd.printf("IR OK");
wait(1);
} else {
lcd.cls();
lcd.printf("IR Fail");
wait(1);
}
// 开始脉搏检测
lcd.cls();
lcd.printf("Pulse Test");
pulseCount = 0;
ticker.attach(&pulseCountCallback, 0.001);
wait(10);
ticker.detach();
// 显示脉搏数
displayPulseCount();
}
}
```
阅读全文
相关推荐
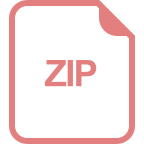





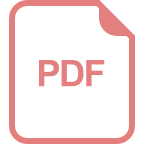
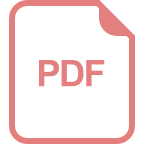
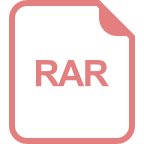
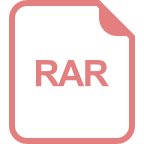
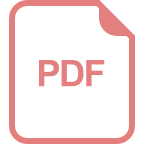





