C语言实现删除指定目录下所有文件
时间: 2024-04-11 17:33:40 浏览: 11
你可以使用C语言的标准库函数和系统调用来实现删除指定目录下所有文件的功能。以下是一个简单的实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
void delete_files(const char *dir_path) {
DIR *dir;
struct dirent *entry;
char path[256];
// 打开指定目录
dir = opendir(dir_path);
if (dir == NULL) {
perror("opendir");
return;
}
// 逐个读取目录项
while ((entry = readdir(dir)) != NULL) {
// 忽略当前目录和上级目录
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0)
continue;
// 构建文件路径
snprintf(path, sizeof(path), "%s/%s", dir_path, entry->d_name);
// 获取文件信息
struct stat info;
if (stat(path, &info) != 0) {
perror("stat");
continue;
}
// 判断是否为普通文件
if (S_ISREG(info.st_mode)) {
// 删除文件
if (remove(path) != 0)
perror("remove");
}
// 判断是否为目录
else if (S_ISDIR(info.st_mode)) {
// 递归删除子目录中的文件
delete_files(path);
// 删除空目录
if (rmdir(path) != 0)
perror("rmdir");
}
}
closedir(dir);
}
int main() {
const char *dir_path = "/path/to/directory"; // 指定目录路径
delete_files(dir_path);
return 0;
}
```
上述代码中的`delete_files`函数用于删除指定目录下的所有文件。它首先打开目录,然后逐个读取目录项。对于每个目录项,它判断是否为普通文件,如果是则直接删除;如果是子目录,则递归调用`delete_files`函数删除子目录中的文件,并最后删除空目录。
请注意,在使用该代码时,你需要将`/path/to/directory`替换为你要删除文件的目录路径。此外,为了安全起见,请务必仔细检查目录路径,确保删除的是正确的目录。
相关推荐
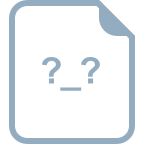
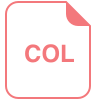
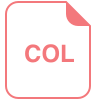
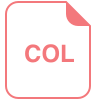
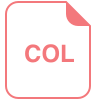
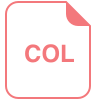









