flask influxdb 连接task数据据实现增删改查的功能
时间: 2023-12-09 10:03:07 浏览: 30
要在Flask中连接InfluxDB并实现增删改查功能,请按照以下步骤操作:
1. 安装influxdb-python库
```
pip install influxdb
```
2. 在Flask应用程序中导入influxdb库
```
from influxdb import InfluxDBClient
```
3. 创建InfluxDBClient对象
```
client = InfluxDBClient(host='localhost', port=8086, username='your_username', password='your_password', database='your_database')
```
4. 实现查询功能
```
def query_data():
result = client.query('select * from your_measurement')
return result.raw['series'][0]['values']
```
5. 实现插入功能
```
def insert_data():
json_body = [
{
"measurement": "your_measurement",
"tags": {
"tag1": "value1",
"tag2": "value2"
},
"time": "2022-01-01T00:00:00Z",
"fields": {
"field1": 1.0,
"field2": 2.0
}
}
]
client.write_points(json_body)
```
6. 实现更新功能
```
def update_data():
client.write_points([
{
"measurement": "your_measurement",
"tags": {"tag1": "value1", "tag2": "value2"},
"time": "2022-01-01T00:00:00Z",
"fields": {"field1": 3.0, "field2": 4.0}
}
], time_precision='s')
```
7. 实现删除功能
```
def delete_data():
client.query('delete from your_measurement where tag1=\'value1\' and time=\'2022-01-01T00:00:00Z\'')
```
以上就是在Flask中连接InfluxDB并实现增删改查功能的基本步骤。在实际应用中,你需要根据自己的需求进行修改和优化。
相关推荐
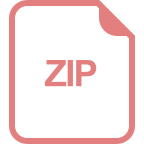
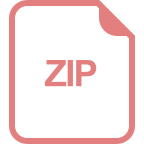














