c语言找出输入次数最多的字符
时间: 2024-10-19 22:10:53 浏览: 20
在C语言中,可以使用哈希表(如`std::unordered_map`)来找出输入字符串中出现次数最多的字符,时间复杂度为线性,远低于O(n^2)。以下是一个简单的实现:
```c
#include <iostream>
#include <unordered_map>
#include <string>
// 函数定义
char findMostFrequentChar(const std::string& str) {
std::unordered_map<char, int> charCount;
// 统计每个字符出现的次数
for (char c : str) {
if (isalnum(c)) { // 检查字符是否为字母或数字
charCount[c]++;
}
}
// 找出出现次数最多的字符
char mostFrequentChar;
int maxCount = 0;
for (const auto& pair : charCount) {
if (pair.second > maxCount) {
mostFrequentChar = pair.first;
maxCount = pair.second;
}
}
return mostFrequentChar;
}
int main() {
std::string input = "aabbbcddddeeffffghijklmnopqrst";
char result = findMostFrequentChar(input);
std::cout << "The character with the highest frequency is: " << result << " and it appears " << maxCount << " times.\n"; // 假设maxCount已计算
return 0;
}
```
阅读全文
相关推荐
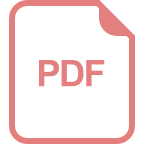
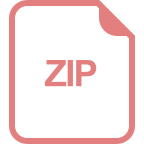
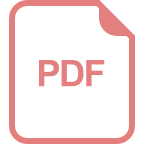















