如何用C语言编程找出一段文本中出现次数最多的字母?
时间: 2024-11-22 18:40:02 浏览: 6
在C语言中,找出一段文本中出现次数最多的字母可以按照以下几个步骤操作:
1. **初始化**:创建一个字符数组(通常是大小为26的数组,因为英文只有26个字母)来存储每个字母及其计数。将所有字母的计数初始化为0。
2. **读取文本**:读取用户输入的字符串,并将其转换为小写,以便不区分大小写。
3. **遍历文本**:遍历文本中的每一个字符,如果它是一个字母(通过检查ASCII码范围),就更新相应的计数。同时,你可以用一个变量记录当前找到的最大计数值和对应的那个字母。
4. **统计**:遍历结束后,遍历字符计数数组,找出最大值以及对应的字母。
5. **返回结果**:最后输出出现次数最多的字母。
这是一个简单的示例代码片段:
```c
#include <stdio.h>
#include <ctype.h>
char alphabet[26] = {0};
int max_count = 0;
char most_frequent_char;
void count_chars(char *text) {
for (int i = 0; text[i]; i++) {
if (isalpha(text[i])) {
text[i] = tolower(text[i]);
alphabet[text[i] - 'a']++;
if (alphabet[text[i] - 'a'] > max_count) {
max_count = alphabet[text[i] - 'a'];
most_frequent_char = text[i];
}
}
}
}
int main() {
char input[100];
printf("Enter a string: ");
fgets(input, sizeof(input), stdin);
count_chars(input);
printf("The character with the highest frequency is '%c' and it appears %d times.\n", most_frequent_char, max_count);
return 0;
}
```
阅读全文
相关推荐
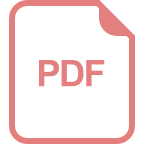
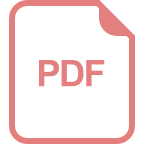
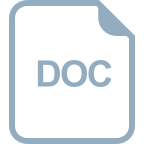
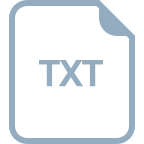
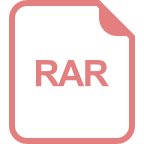
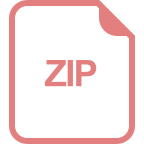
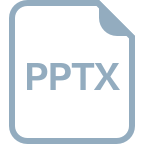
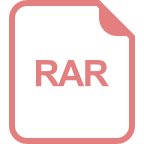
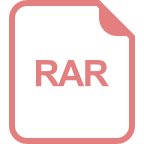
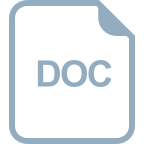
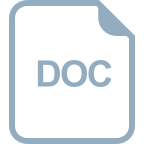
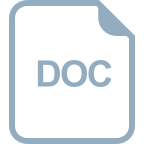
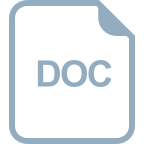
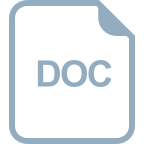
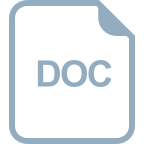
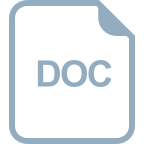
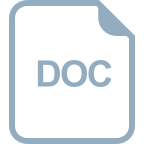
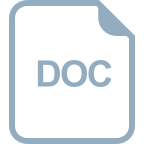
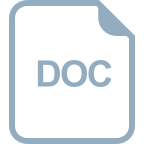