feature_importance = model.get_fscore() feature_importance = sorted(feature_importance.items(), key=lambda d: d[1], reverse=True) feature_importance print(df_columns) dvalid = xgb.DMatrix(valid_X, feature_names=df_columns) predict_valid = model.predict(dvalid) predict_price = np.expm1(predict_valid) valid_true_price = np.expm1(valid_Y) print('决策树模型在验证集上的均方误差 RMSE 为:', rmse(valid_Y, predict_valid))
时间: 2023-06-16 14:06:09 浏览: 49
这段代码使用了XGBoost模型,其中`model.get_fscore()`返回每个特征的重要性评分,然后将其按照评分从大到小进行排序。`df_columns`是特征名列表,`xgb.DMatrix`将验证集转换为DMatrix格式,`model.predict`用于预测验证集的目标值。最后通过指数函数`np.expm1`将预测值和真实值转换为原始价格。`rmse`函数用于计算均方根误差(RMSE)。
相关问题
sorted_idx = perm_importance.importances_mean.argsort()[::-1]是什么意思
这行代码的作用是获取特征重要性的平均值,并将其从大到小排序。具体解释如下:
- importances_mean: 该变量是特征重要性的平均值,代表了每个特征对于模型预测的重要程度。
- argsort(): 该函数会返回数组中元素从小到大排序后的索引值,例如[3, 1, 4, 2]会返回[1, 3, 0, 2],即1对应的值最小,3对应的值次小,以此类推。
- [::-1]: 这个切片操作是将排序后的索引值倒序排列,即从大到小排列。这样排列后,sorted_idx中第一个元素对应的特征重要性是最大的,第二个元素对应的特征重要性次大,以此类推。
因此,sorted_idx是一个一维数组,其中包含了特征重要性从大到小排列后的特征索引值。
from sklearn.preprocessing import StandardScaler scaler = StandardScaler() scaler.fit(X) X_s= scaler.transform(X) X_s[:3] from sklearn.ensemble import RandomForestRegressor model = RandomForestRegressor(n_estimators=5000, max_features=int(X.shape[1] / 3), random_state=0) model.fit(X_s,y) model.score(X_s,y) pred = model.predict(X_s) plt.scatter(pred, y, alpha=0.6) w = np.linspace(min(pred), max(pred), 100) plt.plot(w, w) plt.xlabel('pred') plt.ylabel('y_test') plt.title('Comparison of GDP fitted value and true value') print(model.feature_importances_) sorted_index = model.feature_importances_.argsort() plt.barh(range(X.shape[1]), model.feature_importances_[sorted_index]) plt.yticks(np.arange(X.shape[1]),X.columns[sorted_index],fontsize=14) plt.xlabel('X Importance',fontsize=12) plt.ylabel('covariate X',fontsize=12) plt.title('Importance Ranking Plot of Covariate ',fontsize=15) plt.tight_layout()
这段代码是用于特征标准化、随机森林回归模型训练、模型评估和特征重要性可视化的代码。
首先,通过导入`StandardScaler`库,使用`fit`方法对特征`X`进行标准化处理,然后使用`transform`方法对特征进行转换得到`X_s`。
接着,导入`RandomForestRegressor`库,创建一个包含5000个决策树的随机森林回归模型,其中`n_estimators`表示决策树的数量,`max_features`表示每棵树使用的最大特征数量(这里设置为特征数量的1/3),`random_state`为随机种子。通过调用模型的`fit`方法,使用标准化后的特征`X_s`和目标变量`y`进行训练。
然后,使用训练好的模型对标准化后的特征`X_s`进行预测,得到预测结果`pred`。接着,通过`plt.scatter`绘制预测值和真实值的散点图,并使用`np.linspace`生成一系列数值作为横轴,并绘制一条直线表示预测值和真实值相等的情况。
接下来,通过`print(model.feature_importances_)`打印出特征重要性的值,并使用`argsort()`方法对特征重要性进行排序得到索引。然后使用`plt.barh`绘制水平条形图,横轴表示特征重要性的值,纵轴表示特征的名称,以可视化特征重要性的排名。
最后,通过`plt.tight_layout()`方法调整图像布局,使得图像更加美观。请确保已经导入了相关的库,并将代码中的`X`和`y`替换为实际的数据。
相关推荐
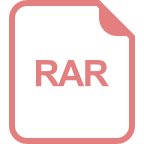












