ax.fill_between(train_sizes, train_scores_mean - train_scores_std, train_scores_mean + train_scores_std, alpha=0.1, color="r") ax.fill_between(train_sizes, test_scores_mean - test_scores_std, test_scores_mean + test_scores_std, alpha=0.1, color="g")
时间: 2024-04-09 15:31:17 浏览: 125
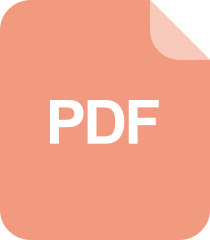
使用sklearn的cross_val_score进行交叉验证实例
这段代码是用于在图形中填充两个区域。第一个区域是训练集得分的平均值减去训练集得分的标准差和训练集得分的平均值加上训练集得分的标准差之间的区域,填充的颜色为红色。第二个区域是测试集得分的平均值减去测试集得分的标准差和测试集得分的平均值加上测试集得分的标准差之间的区域,填充的颜色为绿色。这样可以直观地显示出训练集和测试集得分的差异范围。
阅读全文
相关推荐
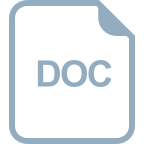
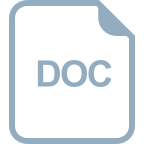














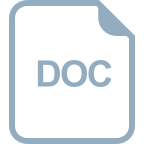
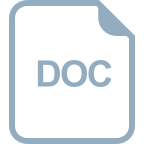