import sys, urllib, urllib.request, os.path def download(url, num_retries=2): print('Download:', url) try: html = urllib.request.urlopen(url).read() except urllib.URLError as e: print('Download error:', e.reason) html = None if num_retries > 0: if hasattr(e, 'code') and 500 <= e.code < 600: return download(url, num_retries-1) return html def main(): url = input('Enter a url:\n'.strip()) f2 = input('Enter a target file:\n'.strip()) if os.path.isfile(f2): print(f2 + 'already exists') sys.exit() html = download(url) target = open(f2, 'w') content = html.decode(encoding='utf-8') target.write(content) target.close()
时间: 2024-02-14 17:06:34 浏览: 18
这是一个 Python 的代码,它定义了一个函数 download() 和一个主函数 main()。download() 函数用于下载 URL 中的内容,如果下载失败则会自动重试,最多重试 2 次。main() 函数会提示用户输入一个 URL 和一个文件名,然后调用 download() 函数下载内容,并将内容写入指定的文件中。
不过,这段代码中有一些问题。首先,在 download() 函数中,当下载失败时,应该使用 return 语句结束函数,否则会继续执行后面的代码,导致程序出错。其次,在 main() 函数中,input() 函数的参数中应该有一个空格,否则用户输入的 URL 和文件名会被拼接在一起。最后,在下载内容后,应该检查 html 变量是否为 None,如果是 None 则说明下载失败,此时应该结束程序。
下面是修正后的代码:
```python
import sys
import urllib.request
import os.path
def download(url, num_retries=2):
print('Download:', url)
try:
html = urllib.request.urlopen(url).read()
except urllib.error.URLError as e:
print('Download error:', e.reason)
html = None
if num_retries > 0:
if hasattr(e, 'code') and 500 <= e.code < 600:
return download(url, num_retries-1)
return None
return html
def main():
url = input('Enter a url:\n').strip()
f2 = input('Enter a target file:\n').strip()
if os.path.isfile(f2):
print(f2 + ' already exists')
sys.exit()
html = download(url)
if html is None:
print('Download failed')
sys.exit()
target = open(f2, 'w', encoding='utf-8')
content = html.decode(encoding='utf-8')
target.write(content)
target.close()
if __name__ == '__main__':
main()
```
相关推荐
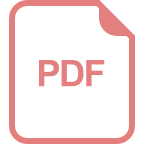
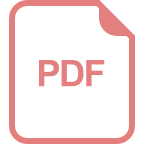
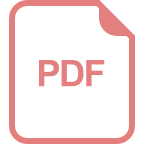













运行下面代码,运行结果没有保存文件,请帮我找出原因 # -- coding: utf-8 -- # 指定文件编码格式为utf-8 import urllib.request import re def getNovertContent(): url = 'http://www.quannovel.com/read/640/' req = urllib.request.Request(url) req.add_header( 'User-Agent', ' Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/113.0.0.0 Safari/537.36') data = urllib.request.urlopen(req).read().decode('gbk') str1 = str(data) # 将网页数据转换为字符串 reg = r'(.?)' reg = re.compile(reg) urls = reg.findall(str1) for url in urls: novel_url = url[0] novel_title = url[1] chapt = urllib.request.urlopen(novel_url).read() chapt_html = chapt.decode('gbk') reg = r'</script> (.?)</script> type="text/javascript">' reg = re.compile(reg, re.S) chapt_content = reg.findall(reg, chapt_html) chapt_content = chapt_content[0].replace( " ", "") chapt_content = chapt_content.replace("
", "") print("正在保存 %s" % novel_title) with open("{}.txt".format(novel_title), 'w') as f: f.write(chapt_content) getNovertContent()

运行下面代码,运行结果没有保存文件,请帮我找出原因 # -- coding: utf-8 -- import urllib.request import re def getNovertContent(): url = 'http://www.quannovel.com/read/640/' req = urllib.request.Request(url) req.add_header('User-Agent', ' Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/113.0.0.0 Safari/537.36') data = urllib.request.urlopen(req).read().decode('gbk') str1 = str(data) # 将网页数据转换为字符串 reg = r'(.?)' reg = re.compile(reg) urls = reg.findall(str1) for url in urls: novel_url = url[0] novel_title = url[1] chapt = urllib.request.urlopen(novel_url).read() chapt_html = chapt.decode('gbk') reg = r'</script> (.?)</script type="text/javascript">' reg = re.compile(reg, re.S) chapt_content = reg.findall(chapt_html) chapt_content = chapt_content[0].replace( " ", "") chapt_content = chapt_content.replace("
", "") print("正在保存 %s" % novel_title) with open("{}.txt".format(novel_title), 'w', encoding='utf-8') as f: f.write(chapt_content) getNovertContent()
