C语言怎么用sort函数对结构体里边的函数进行排序
时间: 2024-05-14 14:15:08 浏览: 61
假设有以下结构体:
```c
struct student {
int id;
char name[20];
int score;
};
```
我们可以用以下代码对结构体数组按照成绩从高到低排序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct student {
int id;
char name[20];
int score;
};
int compare(const void *a, const void *b) {
struct student *s1 = (struct student *)a;
struct student *s2 = (struct student *)b;
return s2->score - s1->score;
}
int main() {
struct student students[] = {
{1, "Tom", 90},
{2, "Jerry", 80},
{3, "Amy", 95},
{4, "Bob", 85}
};
int n = sizeof(students) / sizeof(struct student);
qsort(students, n, sizeof(struct student), compare);
for (int i = 0; i < n; i++) {
printf("%d %s %d\n", students[i].id, students[i].name, students[i].score);
}
return 0;
}
```
在该代码中,我们定义了一个 `compare` 函数作为 `qsort` 函数的比较函数,该函数返回两个结构体比较的结果。然后在 `main` 函数中调用 `qsort` 函数对结构体数组进行排序。最后输出排序后的结果。
阅读全文
相关推荐
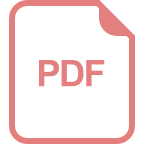
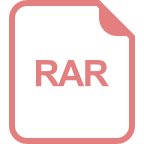

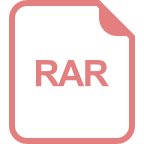
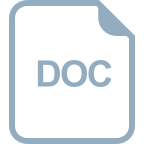
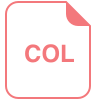










