PSO算法、蚁群算法和遗传算法C++求解函数最值问题,程序怎么编写?
时间: 2024-02-09 09:11:45 浏览: 18
PSO算法、蚁群算法和遗传算法都是优化算法,可以用于解决函数最值问题。下面是各个算法的C++代码示例:
PSO算法:
```C++
#include <iostream>
#include <vector>
#include <random>
#include <cmath>
using namespace std;
default_random_engine generator;
uniform_real_distribution<double> distribution(0.0, 1.0);
double f(vector<double> x) {
// 计算适应度函数值
double sum = 0;
for (int i = 0; i < x.size(); i++) {
sum += pow(x[i], 2);
}
return sum;
}
vector<double> PSO(int nvars, vector<double> lb, vector<double> ub, int maxiter, int swarmsize, double c1, double c2, double w) {
vector<vector<double>> x(swarmsize, vector<double>(nvars));
vector<vector<double>> v(swarmsize, vector<double>(nvars));
vector<vector<double>> pbest(swarmsize, vector<double>(nvars));
vector<double> pbestval(swarmsize);
vector<double> gbest(nvars);
double gbestval = INFINITY;
// 初始化
for (int i = 0; i < swarmsize; i++) {
for (int j = 0; j < nvars; j++) {
x[i][j] = lb[j] + (ub[j] - lb[j]) * distribution(generator);
v[i][j] = 0;
}
pbest[i] = x[i];
pbestval[i] = f(x[i]);
if (pbestval[i] < gbestval) {
gbestval = pbestval[i];
gbest = pbest[i];
}
}
// 迭代
for (int iter = 0; iter < maxiter; iter++) {
for (int i = 0; i < swarmsize; i++) {
for (int j = 0; j < nvars; j++) {
// 更新速度
v[i][j] = w * v[i][j] + c1 * distribution(generator) * (pbest[i][j] - x[i][j]) + c2 * distribution(generator) * (gbest[j] - x[i][j]);
// 更新位置
x[i][j] = x[i][j] + v[i][j];
// 边界处理
if (x[i][j] < lb[j]) {
x[i][j] = lb[j];
}
if (x[i][j] > ub[j]) {
x[i][j] = ub[j];
}
}
// 更新个体最优值
double fx = f(x[i]);
if (fx < pbestval[i]) {
pbest[i] = x[i];
pbestval[i] = fx;
// 更新群体最优值
if (pbestval[i] < gbestval) {
gbestval = pbestval[i];
gbest = pbest[i];
}
}
}
// 更新惯性权重
w = w * 0.99;
}
return gbest;
}
```
蚁群算法:
```C++
#include <iostream>
#include <vector>
#include <random>
#include <cmath>
using namespace std;
default_random_engine generator;
uniform_real_distribution<double> distribution(0.0, 1.0);
double f(vector<int> x) {
// 计算适应度函数值
double sum = 0;
for (int i = 0; i < x.size(); i++) {
sum += pow(x[i], 2);
}
return sum;
}
vector<int> AntColony(int nvars, vector<int> lb, vector<int> ub, int maxiter, int antsize, double alpha, double beta, double rho, double q0) {
vector<vector<int>> x(antsize, vector<int>(nvars));
vector<double> fitness(antsize);
vector<int> bestx(nvars);
double bestfval = INFINITY;
vector<vector<double>> pheromone(nvars, vector<double>(nvars, 1.0 / (nvars * nvars)));
// 迭代
for (int iter = 0; iter < maxiter; iter++) {
// 移动蚂蚁
for (int i = 0; i < antsize; i++) {
x[i][0] = lb[0] + round(distribution(generator) * (ub[0] - lb[0]));
for (int j = 1; j < nvars; j++) {
vector<double> prob(nvars);
vector<int> visited(nvars);
visited[x[i][j - 1]] = 1;
for (int k = 0; k < nvars; k++) {
if (!visited[k]) {
prob[k] = pow(pheromone[x[i][j - 1]][k], alpha) * pow(1.0 / abs(k - x[i][j - 1]), beta);
}
}
double randval = distribution(generator);
if (randval < q0) {
double maxval = -INFINITY;
int maxidx = -1;
for (int k = 0; k < nvars; k++) {
if (prob[k] > maxval) {
maxval = prob[k];
maxidx = k;
}
}
x[i][j] = maxidx;
}
else {
double sumprob = 0;
for (int k = 0; k < nvars; k++) {
sumprob += prob[k];
}
for (int k = 0; k < nvars; k++) {
prob[k] /= sumprob;
}
double randval2 = distribution(generator);
double cumprob = 0;
for (int k = 0; k < nvars; k++) {
cumprob += prob[k];
if (randval2 < cumprob) {
x[i][j] = k;
break;
}
}
}
}
// 更新最优解
double fx = f(x[i]);
if (fx < bestfval) {
bestx = x[i];
bestfval = fx;
}
}
// 更新信息素
vector<vector<double>> delta_pheromone(nvars, vector<double>(nvars));
for (int i = 0; i < antsize; i++) {
for (int j = 0; j < nvars - 1; j++) {
delta_pheromone[x[i][j]][x[i][j + 1]] += 1.0 / f(x[i]);
}
}
for (int i = 0; i < nvars; i++) {
for (int j = 0; j < nvars; j++) {
pheromone[i][j] = (1 - rho) * pheromone[i][j] + delta_pheromone[i][j];
}
}
}
return bestx;
}
```
遗传算法:
```C++
#include <iostream>
#include <vector>
#include <random>
#include <cmath>
using namespace std;
default_random_engine generator;
uniform_real_distribution<double> distribution(0.0, 1.0);
double f(vector<double> x) {
// 计算适应度函数值
double sum = 0;
for (int i = 0; i < x.size(); i++) {
sum += pow(x[i], 2);
}
return sum;
}
vector<double> GeneticAlgorithm(int nvars, vector<double> lb, vector<double> ub, int maxgenerations, int popsize, double mutationrate, double crossoverfraction) {
vector<vector<double>> pop(popsize, vector<double>(nvars));
vector<double> fitness(popsize);
vector<double> bestx(nvars);
double bestfval = INFINITY;
// 初始化
for (int i = 0; i < popsize; i++) {
for (int j = 0; j < nvars; j++) {
pop[i][j] = lb[j] + (ub[j] - lb[j]) * distribution(generator);
}
fitness[i] = f(pop[i]);
if (fitness[i] < bestfval) {
bestfval = fitness[i];
bestx = pop[i];
}
}
// 迭代
for (int gen = 0; gen < maxgenerations; gen++) {
// 选择
vector<vector<double>> parents(popsize, vector<double>(nvars));
vector<double> parentsfitness(popsize);
for (int i = 0; i < popsize; i++) {
int idx1 = rand() % popsize;
int idx2 = rand() % popsize;
if (fitness[idx1] < fitness[idx2]) {
parents[i] = pop[idx1];
parentsfitness[i] = fitness[idx1];
}
else {
parents[i] = pop[idx2];
parentsfitness[i] = fitness[idx2];
}
}
// 交叉
vector<vector<double>> children;
for (int i = 0; i < popsize / 2; i++) {
int idx1 = rand() % popsize;
int idx2 = rand() % popsize;
vector<double> parent1 = parents[idx1];
vector<double> parent2 = parents[idx2];
vector<double> child1(nvars);
vector<double> child2(nvars);
for (int j = 0; j
相关推荐
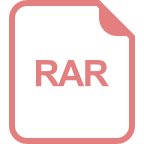
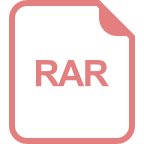














