python车速检测代码
时间: 2023-09-17 09:07:18 浏览: 113
以下是一个简单的Python车速检测代码,使用OpenCV库:
```python
import cv2
import numpy as np
# 读取视频文件
cap = cv2.VideoCapture('video.avi')
# 初始化变量
frame_count = 0
last_speed = 0
# 设置感兴趣区域
roi = [(580, 460), (700, 460), (1040, 720), (0, 720)]
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
# 根据ROI裁剪图像
mask = np.zeros_like(frame)
cv2.fillPoly(mask, [np.array(roi)], (255, 255, 255))
cropped_frame = cv2.bitwise_and(frame, mask)
# 转换为灰度图像
gray = cv2.cvtColor(cropped_frame, cv2.COLOR_BGR2GRAY)
# 应用高斯模糊
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
# 应用Canny边缘检测
edges = cv2.Canny(blurred, 50, 150)
# 应用霍夫变换检测直线
lines = cv2.HoughLinesP(edges, 1, np.pi/180, 50, minLineLength=50, maxLineGap=10)
# 计算车速
if lines is not None:
for line in lines:
x1, y1, x2, y2 = line[0]
# 判断直线是否在ROI内部
if y1 >= roi[0][1] and y2 >= roi[0][1]:
# 计算直线长度
length = np.sqrt((x2-x1)**2 + (y2-y1)**2)
# 计算车速(假设每个像素代表0.1米)
speed = length / 100 * 25 * 60
# 平滑车速值
if frame_count == 0:
last_speed = speed
else:
last_speed = 0.9 * last_speed + 0.1 * speed
# 在图像上显示车速值
cv2.putText(cropped_frame, "Speed: {:.2f} km/h".format(last_speed), (10, 50), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
# 显示视频帧
cv2.imshow('frame', cropped_frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
frame_count += 1
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
这个代码使用了Canny边缘检测和霍夫变换检测直线,然后根据直线长度计算车速。你可以根据自己的需求进行修改。
相关推荐
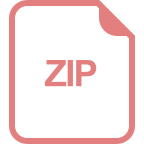














