You are given two arrays a and b , both of length n . Your task is to count the number of pairs of integers (i,j) such that 1≤i<j≤n and ai⋅aj=bi+bj .
时间: 2024-02-06 07:02:54 浏览: 149
To solve this problem, we can first create a dictionary to store the count of each value in array `b`. Then, we can loop through all pairs of indices `(i,j)` and check if `a[i]*a[j]` is equal to `b[i]+b[j]`. If it is, we increment our count by the number of times `b[i]+b[j]` appears in our dictionary.
Here's some sample code in Python:
```python
def count_pairs(a, b):
n = len(a)
b_count = {}
for num in b:
b_count[num] = b_count.get(num, 0) + 1
pair_count = 0
for i in range(n):
for j in range(i+1, n):
if a[i]*a[j] == b[i]+b[j]:
pair_count += b_count.get(b[i]+b[j], 0)
return pair_count
```
Note that we use the `get` method of dictionaries to handle the case where `b[i]+b[j]` is not in our dictionary.
阅读全文
相关推荐
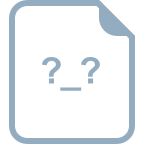
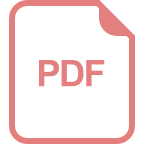
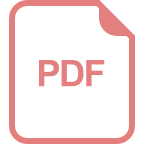

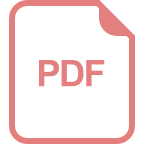
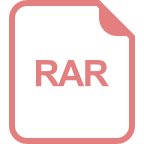
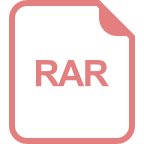
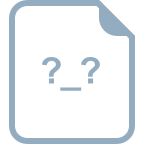
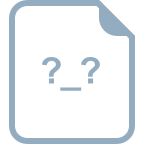
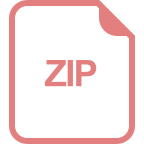
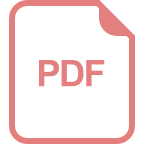
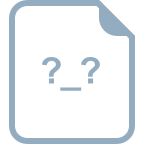
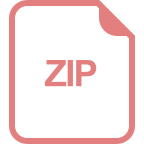
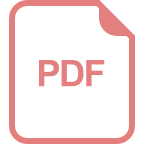
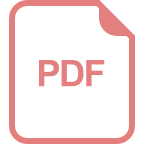