You are given two arrays a and b,both of length n Your task is to count the number of pairs of integers (i,j) such that 1≤i<j≤n and ai⋅aj=bi+bj .
时间: 2024-02-06 11:02:54 浏览: 116
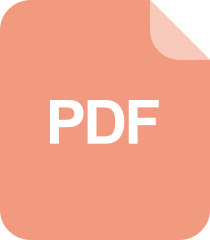
A computationally efficient source localization method for a mixture of near-field and far-field narrowband signals
To count the number of pairs of integers (i,j) such that ai⋅aj=bi+bj, we can use a hash table to keep track of the count of each value in array a and b. Then, we can loop through all pairs of indices (i,j) and check if ai⋅aj=bi+bj. If it is true, we increment a counter.
Here is the code:
```
def count_pairs(a, b):
n = len(a)
count_a = {}
count_b = {}
for i in range(n):
count_a[a[i]] = count_a.get(a[i], 0) + 1
count_b[b[i]] = count_b.get(b[i], 0) + 1
count = 0
for i in range(n):
for j in range(i+1, n):
if a[i]*a[j] == b[i]+b[j]:
count += 1
return count
```
Note that this solution has a time complexity of O(n^2) because of the nested loops. However, we can make it more efficient by using a set to store all possible values of ai⋅aj and bi+bj, and then check if each product is in the set. This reduces the time complexity to O(n).
阅读全文
相关推荐
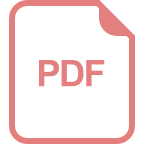
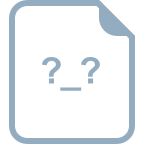

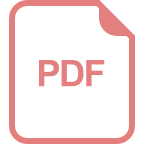
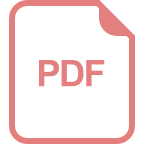
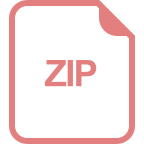
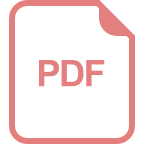
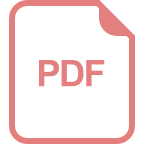
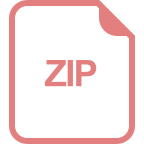
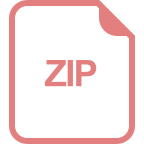
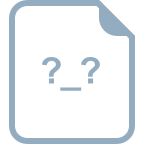
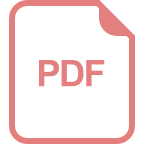
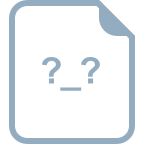
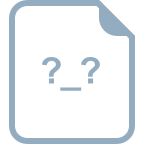
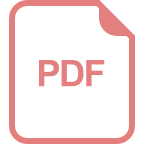