STM32串口打印一段时间后自己停止什么原因
时间: 2024-05-22 12:16:46 浏览: 12
有可能是以下原因导致STM32串口打印一段时间后自己停止:
1. 缓存溢出:当串口接收数据的速度大于处理数据的速度时,数据会在缓存中积累,最终导致缓存溢出。如果缓存溢出,数据就会丢失,导致串口停止打印。
2. 电源问题:如果STM32的电源不稳定,可能会导致串口停止打印。这可能是由于电源波动或电源噪声引起的。
3. 代码错误:如果程序中有逻辑错误或死循环,可能会导致串口停止打印。在这种情况下,需要检查代码并修复错误。
4. 硬件问题:如果串口接收器或发送器存在问题,可能会导致串口停止打印。在这种情况下,需要检查硬件并修复问题。
5. 波特率设置错误:如果波特率设置错误,可能会导致串口停止打印。在这种情况下,需要检查波特率设置并进行更正。
相关问题
STM32串口接收一段数据怎么写程序
在STM32上实现串口接收一段数据,可以通过以下步骤实现:
1. 配置串口:在STM32的库函数中,可以使用USART_Init函数初始化串口,设置波特率、数据位、停止位和校验位等参数。通过USART_Cmd函数使能串口。
2. 配置中断:USART_ITConfig函数可以设置串口中断,使得每当串口接收到数据时,就会触发中断。可以使用NVIC_Init函数初始化中断。
3. 编写中断服务函数:在中断服务函数中,通过USART_ReceiveData函数读取接收到的数据,可以使用一个缓冲区来存储接收到的数据,以便后续处理。
4. 处理接收到的数据:在串口接收到数据后,可以在中断服务函数中处理数据,例如将数据存储到数组中、进行校验、打印输出等操作。
以下是一个使用STM32库函数实现串口接收的示例代码:
```
#include "stm32f10x.h"
#include <stdio.h>
#define BUFFER_SIZE 10 //定义缓冲区大小
uint8_t Buffer[BUFFER_SIZE]; //定义一个缓冲区
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
//使能串口和GPIO时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
//配置串口引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
//配置串口参数
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
//使能串口中断
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
//使能中断
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
//使能串口
USART_Cmd(USART1, ENABLE);
while (1)
{
//处理接收到的数据
//...
}
}
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET)
{
static uint8_t i = 0;
Buffer[i++] = USART_ReceiveData(USART1);
if (i == BUFFER_SIZE)
{
i = 0;
//处理接收到的数据
//...
}
}
}
```
以上代码中,我们使用USART1作为串口,并在GPIOA9和GPIOA10引脚上进行配置。在中断服务函数USART1_IRQHandler中,使用static关键字定义一个i变量,每次接收到数据时将数据存储到缓冲区中,并在缓冲区满时进行数据处理。在while(1)循环中,可以对接收到的数据进行处理。
stm32串口通讯异常处理
### 回答1:
在STM32中,串口通讯异常处理可以分为硬件异常和软件异常两种情况。
硬件异常:
1. 帧错误(FE):由于信号线上的噪声或其他因素导致数据位接收错误,此时会触发帧错误。可以通过串口驱动中的帧错误中断来处理。
2. 奇偶校验错误(PE):数据在传输时进行奇偶校验,如果校验结果与预期不符,则会触发奇偶校验错误。可以通过串口驱动中的奇偶校验错误中断来处理。
3. 越界错误(ORE):当接收数据缓冲区溢出时,会触发越界错误。可以通过串口驱动中的越界错误中断来处理。
软件异常:
1. 接收超时:当串口接收数据时,如果一段时间内没有接收到新的数据,则会触发接收超时。可以通过设置串口接收超时时间和使用串口驱动中的接收超时中断来处理。
2. 发送失败:当串口发送数据时,如果发送失败,则需要重新发送。可以通过设置串口发送超时时间和使用串口驱动中的发送失败中断来处理。
在处理异常时,可以记录异常信息并进行相应的处理,例如重新打开串口或输出调试信息。
### 回答2:
STM32串口通讯时可能出现的异常有以下几种情况:
1. 丢失数据:在传输过程中,由于硬件原因或者程序错误,可能会导致数据丢失。处理该异常的方法是,增加数据校验机制,如使用校验和或CRC校验来保证数据的完整性。同时,可以设置数据重传机制,当接收端检测到数据丢失时,可以要求发送端重新发送数据。
2. 帧错误:数据传输过程中,可能由于干扰、电压不稳定等原因,导致数据帧出错。处理该异常的方法是,在接收端使用帧同步机制,确保每个数据帧开始和结束的标志位正确。在发送端,可以使用硬件流控制或者软件延时等方式,确保数据帧的发送顺序和时间间隔。
3. 超时:在某些情况下,可能会由于噪声、线路故障等原因,导致串口通讯超时。处理该异常的方法是,在接收端设置超时计时器,如果在规定时间内没有接收到数据,则重新发送请求或者中断通讯。
4. 缓冲区溢出:由于数据传输速度过快,接收端的缓冲区可能会溢出。处理该异常的方法是,增加缓冲区大小,或者使用中断方式接收数据,确保能够及时处理数据。同时,可以设置数据流控制,当接收端的处理能力不足时,向发送端发送停止发送的信号。
在进行STM32串口通讯时,需要根据不同的应用场景和需求,采取适当的异常处理方法。通过合理的设计和实施,可以确保串口通讯的稳定性和可靠性。
### 回答3:
stm32串口通讯异常处理是指在使用stm32单片机进行串口通信时,当遇到通信异常情况时所需要采取的处理措施。
首先,我们需要检查串口通信的硬件连接是否正确,包括连接线是否接触良好、是否存在短路或接地问题等。如果存在硬件故障,则需要修复或更换硬件元件。
其次,我们需要检查串口通信的软件配置是否正确。这包括设置波特率、数据位、校验位和停止位等参数。如果发现设置有误,需要及时进行修正。
在进行串口通信时,还要注意数据的发送和接收过程。我们可以通过在发送数据前进行数据的检验和校验等方式,来避免发送无效的数据。同时,还要根据接收的数据进行校验,判断接收数据的完整性和正确性。如果发现接收到的数据不正确,可以进行重传或请求补发等操作。
此外,也可以通过在编程代码中添加错误处理机制,当发生异常情况时,能够及时捕获并进行处理。例如,可以在代码中添加超时检测机制,如果在规定时间内没有接收到数据,则判断为异常情况。
最后,为了更好地调试和排查串口通信异常问题,我们可以添加调试信息输出功能,将相关信息打印在串口终端,以便进行分析和定位问题。
综上所述,对于stm32串口通信异常处理,我们需要从硬件连线、软件配置、数据的发送接收和错误处理等方面进行全面而细致的分析和处理,以确保串口通信的稳定与可靠。
相关推荐
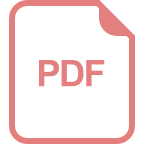












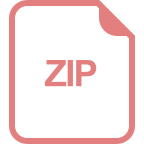