你能用C语言编写一个功能丰富的贪吃蛇游戏吗
时间: 2024-05-16 20:13:03 浏览: 54
是的,我可以使用C语言编写一个贪吃蛇游戏。以下是一个简单的示例代码,可以让你开始学习如何使用C语言编写贪吃蛇游戏。
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#include <windows.h>
#define ROW 20
#define COL 20
int score = 0; //得分
int speed = 200; //速度
int snake_len = 0; //蛇的长度
//定义坐标结构体
typedef struct {
int x;
int y;
} location;
location food; //食物坐标
location snake[ROW * COL]; //蛇的每一节身体的坐标
//初始化蛇的位置
void init_snake() {
snake[0].x = ROW / 2;
snake[0].y = COL / 2;
snake_len++;
}
//初始化食物的位置
void init_food() {
srand((unsigned)time(NULL));
food.x = rand() % ROW;
food.y = rand() % COL;
}
//绘制游戏界面
void draw() {
system("cls");
printf("Score: %d\n", score);
for (int i = 0; i < ROW + 2; i++) {
printf("#");
}
printf("\n");
for (int i = 0; i < ROW; i++) {
printf("#");
for (int j = 0; j < COL; j++) {
if (i == snake[0].x && j == snake[0].y) {
printf("O");
} else if (i == food.x && j == food.y) {
printf("F");
} else {
int flag = 0;
for (int k = 1; k < snake_len; k++) {
if (i == snake[k].x && j == snake[k].y) {
printf("o");
flag = 1;
break;
}
}
if (!flag) {
printf(" ");
}
}
}
printf("#\n");
}
for (int i = 0; i < ROW + 2; i++) {
printf("#");
}
printf("\n");
}
//移动蛇
void move_snake(int direction) {
//判断是否撞墙
if (snake[0].x < 0 || snake[0].x >= ROW || snake[0].y < 0 || snake[0].y >= COL) {
printf("Game over!\n");
exit(0);
}
//判断是否吃到食物
if (snake[0].x == food.x && snake[0].y == food.y) {
score++;
speed -= 10;
init_food();
snake_len++;
}
//移动蛇身
for (int i = snake_len - 1; i > 0; i--) {
snake[i].x = snake[i - 1].x;
snake[i].y = snake[i - 1].y;
}
//根据方向移动蛇头
switch (direction) {
case 'w':
snake[0].x--;
break;
case 'a':
snake[0].y--;
break;
case 's':
snake[0].x++;
break;
case 'd':
snake[0].y++;
break;
}
//判断是否撞到自己的身体
for (int i = 1; i < snake_len; i++) {
if (snake[0].x == snake[i].x && snake[0].y == snake[i].y) {
printf("Game over!\n");
exit(0);
}
}
}
int main() {
init_snake();
init_food();
draw();
while (1) {
if (_kbhit()) {
int direction = _getch();
if (direction == 'w' || direction == 'a' || direction == 's' || direction == 'd') {
move_snake(direction);
}
}
move_snake('s');
draw();
Sleep(speed);
}
return 0;
}
```
这个示例代码中,我们使用了Windows.h头文件中的Sleep函数来控制游戏速度,使用了conio.h头文件中的_kbhit和_getch函数来获取用户键盘输入的方向。你可以根据自己的需要修改代码来使游戏界面更加美观,增加游戏难度等功能。
阅读全文
相关推荐






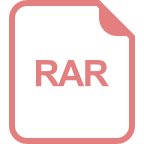










