使用链式结构建立学生信息表,实现建表、插入、删除和打印等基本功能。学生信息包括学号、姓名、成绩。 建表(c):能通过输入指令建立空白学生信息表。 插入(i):能够在指定位置插入一个学生信息。 删除(d):能够删除指定位置的学生信息。 打印(p):能够打印输出完整学生信息表。
时间: 2024-12-01 07:20:21 浏览: 35
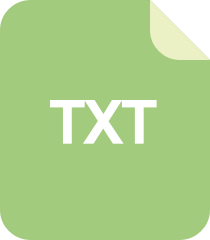
学生信息管理系统的顺序表实现
在C语言中,利用链表结构可以方便地实现一个简单的学生信息表。首先,我们需要定义两个结构体:一个是存储单个学生的`Stu`结构体,包含学号、姓名和成绩;另一个是链表节点`ListNode`,每个节点保存一个`Stu`实例并指向下一个节点。
```c
typedef struct Student {
unsigned char ID[ID_LENGTH]; // 学号
char Name[NAME_LENGTH]; // 姓名
int Score; // 成绩
} Stu;
typedef struct LNode {
Stu student;
struct LNode* next;
} ListNode;
```
然后,我们可以实现四个核心功能:
1. **建表(ListCreation)**
创建一个空链表,表示为空的表。
```c
ListNode* ListCreation() {
ListNode* head = (ListNode*) malloc(sizeof(ListNode)); // 分配内存
head->student.ID[0] = '\0'; // 初始化首节点的学号为空字符串
head->student.Name[0] = '\0';
head->student.Score = 0;
head->next = NULL;
return head;
}
```
2. **插入(ListInsert)**
根据指定位置插入新的学生信息。
```c
void ListInsert(ListNode** head, int pos, Stu newStudent) {
ListNode* newNode = (ListNode*) malloc(sizeof(ListNode));
newNode->student = newStudent;
newNode->next = (*head)->next;
if (pos == 0) { // 插入到头部
(*head) = newNode;
} else {
ListNode* temp = (*head);
for (int i = 0; i < pos - 1 && temp != NULL; ++i) {
temp = temp->next;
}
if (temp != NULL) {
newNode->next = temp->next;
temp->next = newNode;
} else {
printf("插入位置超出范围\n"); // 处理边界情况
}
}
}
```
3. **删除(ListDelete)**
删除指定位置的学生信息。
```c
void ListDelete(ListNode** head, int pos) {
if (*head == NULL) return;
ListNode* current = *head;
ListNode* previous = NULL;
if (pos == 0) {
*head = current->next;
free(current);
} else {
for (int i = 0; i < pos - 1 && current != NULL; ++i) {
previous = current;
current = current->next;
}
if (current != NULL) {
previous->next = current->next;
free(current);
} else {
printf("删除位置超出范围\n");
}
}
}
```
4. **打印(ListPrint)**
打印整个学生信息表。
```c
void ListPrint(ListNode* head) {
if (head == NULL) return;
ListNode* temp = head;
while (temp != NULL) {
printf("学号:%s 姓名:%s 成绩:%d\n",
temp->student.ID, temp->student.Name, temp->student.Score);
temp = temp->next;
}
}
```
使用这些函数时,首先要创建一个表,然后根据需求插入、删除或打印信息。记得处理内存分配和释放,以及边界条件的检查。
阅读全文
相关推荐
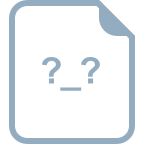
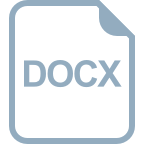






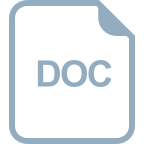
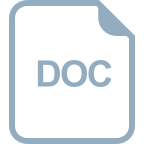
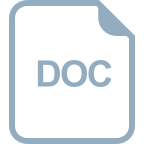





