使用链式结构建立学生信息表,实现建表、插入、删除和打印等基本功能。学生信息包括学号、姓名、成绩。
时间: 2024-11-29 22:32:35 浏览: 24
在数据库设计中,我们可以使用链式结构(比如链表数据结构)来创建一个动态的学生信息表。这里以伪代码的形式展示如何实现这些基本操作:
首先,我们定义一个`Student`节点类,包含学生的属性如学号(id)、姓名(name)和成绩(score):
```python
class StudentNode:
def __init__(self, id, name, score):
self.id = id
self.name = name
self.score = score
self.next = None
class StudentInfoList:
def __init__(self):
self.head = None
```
然后,我们可以实现链式结构的基本操作:
1. **建表**:在这个例子中,“建表”实际上就是初始化一个空的学生列表。
```python
def create_table(self):
self.head = StudentNode(None, None, None)
self.head.next = None
```
2. **插入**:向链表中添加新学生的信息。
```python
def insert_student(self, id, name, score):
new_node = StudentNode(id, name, score)
if not self.head:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
```
3. **删除**:根据学号删除特定的学生。
```python
def delete_student(self, id):
if not self.head:
return
if self.head.id == id:
self.head = self.head.next
return
prev = self.head
current = self.head.next
while current and current.id != id:
prev = current
current = current.next
if current:
prev.next = current.next
```
4. **打印**:遍历链表并打印所有学生的详细信息。
```python
def print_students(self):
current = self.head
while current:
print("学号:", current.id, "姓名:", current.name, "成绩:", current.score)
current = current.next
```
阅读全文
相关推荐
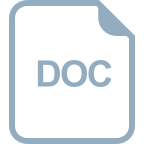
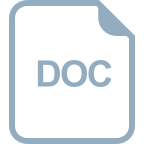
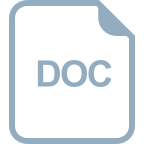


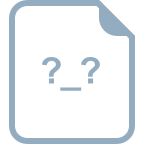





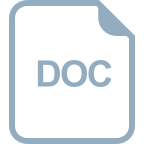






