我问的是最大内接斜矩形怎么求
时间: 2023-09-04 12:11:37 浏览: 72
要获取轮廓的最大内接斜矩形,可以使用OpenCV中的minAreaRect函数。与获取最大内接矩形的方法类似,该函数同样可以返回一个包含轮廓的最小矩形区域,包括矩形的中心点坐标、长宽和旋转角度。具体实现步骤如下:
1. 读取图像并进行灰度转换和二值化处理。
```python
import cv2
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
```
2. 查找轮廓并筛选出最大轮廓。
```python
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
max_contour = max(contours, key=cv2.contourArea)
```
3. 获取最大内接矩形的信息,并绘制出来。
```python
rect = cv2.minAreaRect(max_contour)
box = cv2.boxPoints(rect)
box = np.int0(box)
cv2.drawContours(img, [box], 0, (0, 0, 255), 2)
```
其中,boxPoints函数可以将最小矩形的四个顶点坐标返回为一个数组,np.int0函数可以将浮点数转换为整型。最后,使用drawContours函数将最小矩形绘制在原图上。
完整代码如下:
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
max_contour = max(contours, key=cv2.contourArea)
rect = cv2.minAreaRect(max_contour)
box = cv2.boxPoints(rect)
box = np.int0(box)
cv2.drawContours(img, [box], 0, (0, 0, 255), 2)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
值得注意的是,minAreaRect函数返回的矩形旋转角度是以水平方向为0度的逆时针旋转角度,如果需要将其转换为顺时针旋转角度,则可以使用以下代码进行转换:
```python
angle = rect[2]
if angle < -45:
angle += 90
```
这里将旋转角度小于-45度的矩形转换为顺时针旋转角度。
相关推荐
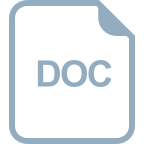
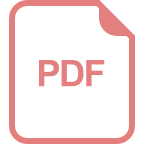
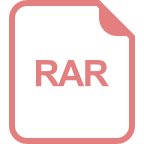














