给出一个使用keras神经网络模型的文本分类代码
时间: 2024-03-10 13:49:43 浏览: 84
以下是一个使用 Keras 模型进行文本分类的示例代码:
```python
from keras.models import Sequential
from keras.layers import Dense, Embedding, LSTM, Dropout
from keras.preprocessing.text import Tokenizer
from keras.preprocessing.sequence import pad_sequences
from keras.utils import np_utils
import numpy as np
# 准备数据
texts = ["I like to play football", "He hates playing video games", "She loves dancing"]
labels = [0, 1, 2] # 0表示“体育”,1表示“游戏”,2表示“音乐”
# 将标签转换为 one-hot 编码
labels = np_utils.to_categorical(labels)
# 构建词汇表
tokenizer = Tokenizer(num_words=1000)
tokenizer.fit_on_texts(texts)
sequences = tokenizer.texts_to_sequences(texts)
word_index = tokenizer.word_index
print('Found %s unique tokens.' % len(word_index))
# 将序列填充到相同的长度
max_sequence_length = max([len(seq) for seq in sequences])
data = pad_sequences(sequences, maxlen=max_sequence_length)
# 构建模型
model = Sequential()
model.add(Embedding(input_dim=len(word_index) + 1, output_dim=256, input_length=max_sequence_length))
model.add(LSTM(256, dropout=0.2, recurrent_dropout=0.2))
model.add(Dense(3, activation='softmax'))
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
# 训练模型
model.fit(data, labels, epochs=10, batch_size=32)
# 使用模型进行预测
test_text = ["She likes to play basketball"]
test_sequence = tokenizer.texts_to_sequences(test_text)
test_data = pad_sequences(test_sequence, maxlen=max_sequence_length)
prediction = model.predict(test_data)
print(prediction)
```
在上面的代码中,我们使用了 Embedding 层将每个单词嵌入到一个 256 维的空间中。然后,我们使用 LSTM 层来学习输入序列的特征。最后,我们将 LSTM 的输出连接到一个大小为 3 的全连接层上,使用 softmax 激活函数进行多分类。在训练模型时,我们使用交叉熵损失函数和 Adam 优化器进行优化。在预测时,我们将测试文本转换为序列并进行填充,然后使用模型进行预测。
阅读全文
相关推荐
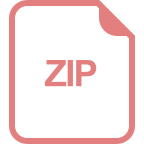
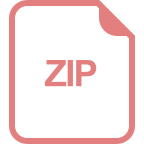
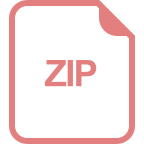















