编制一个能够分析整数、小数、标识符、主要运算符、主要关键字的词法分析器。用C语言实现
时间: 2023-10-03 11:08:28 浏览: 174
以下是一个简单的词法分析器实现,可以识别整数、小数、标识符、主要运算符和主要关键字。该实现基于有限状态自动机(FSM)。
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#define MAX_TOKEN_LENGTH 100
// Token类型
typedef enum {
TOKEN_INTEGER,
TOKEN_FLOAT,
TOKEN_IDENTIFIER,
TOKEN_OPERATOR,
TOKEN_KEYWORD
} TokenType;
// Token结构体
typedef struct {
TokenType type;
char value[MAX_TOKEN_LENGTH];
} Token;
// 关键字列表
const char *keywords[] = {
"if",
"else",
"while",
"for",
"int",
"float",
"double",
"char",
"void"
};
const int num_keywords = 9;
// 判断一个字符串是否是关键字
int is_keyword(char *str) {
for (int i = 0; i < num_keywords; i++) {
if (strcmp(str, keywords[i]) == 0) {
return 1;
}
}
return 0;
}
// 词法分析函数
Token get_next_token(FILE *fp) {
Token token;
char c = fgetc(fp);
token.value[0] = '\0';
// 跳过空格和换行符
while (isspace(c)) {
c = fgetc(fp);
}
// 整数或浮点数
if (isdigit(c)) {
int i = 0;
while (isdigit(c) || c == '.') {
token.value[i++] = c;
c = fgetc(fp);
}
token.value[i] = '\0';
ungetc(c, fp);
if (strchr(token.value, '.') != NULL) {
token.type = TOKEN_FLOAT;
} else {
token.type = TOKEN_INTEGER;
}
return token;
}
// 标识符或关键字
if (isalpha(c) || c == '_') {
int i = 0;
while (isalnum(c) || c == '_') {
token.value[i++] = c;
c = fgetc(fp);
}
token.value[i] = '\0';
ungetc(c, fp);
if (is_keyword(token.value)) {
token.type = TOKEN_KEYWORD;
} else {
token.type = TOKEN_IDENTIFIER;
}
return token;
}
// 运算符
if (c == '+' || c == '-' || c == '*' || c == '/' || c == '%' || c == '=' || c == '<' || c == '>' || c == '&' || c == '|') {
token.type = TOKEN_OPERATOR;
token.value[0] = c;
token.value[1] = '\0';
return token;
}
// 如果无法识别,返回空token
token.type = -1;
return token;
}
int main() {
FILE *fp;
Token token;
fp = fopen("input.txt", "r");
if (fp == NULL) {
printf("Error opening file.\n");
return 1;
}
while ((token = get_next_token(fp)).type != -1) {
switch (token.type) {
case TOKEN_INTEGER:
printf("整数数值:%s\n", token.value);
break;
case TOKEN_FLOAT:
printf("浮点数值:%s\n", token.value);
break;
case TOKEN_IDENTIFIER:
printf("标识符:%s\n", token.value);
break;
case TOKEN_OPERATOR:
printf("运算符:%s\n", token.value);
break;
case TOKEN_KEYWORD:
printf("关键字:%s\n", token.value);
break;
default:
break;
}
}
fclose(fp);
return 0;
}
```
该词法分析器读取一个包含代码的文本文件,并输出识别出的各种token及其值。例如:
```
int main() {
float x = 2.5;
if (x > 0) {
printf("x is positive");
} else {
printf("x is non-positive");
}
}
```
输出:
```
关键字:int
标识符:main
运算符:(
运算符:)
运算符:{
关键字:float
标识符:x
运算符:=
浮点数值:2.5
运算符:;
关键字:if
运算符:(
标识符:x
运算符:>
整数数值:0
运算符:)
运算符:{
标识符:printf
运算符:(
字符串值:"x is positive"
运算符:)
运算符:;
运算符:}
关键字:else
运算符:{
标识符:printf
运算符:(
字符串值:"x is non-positive"
运算符:)
运算符:;
运算符:}
```
阅读全文
相关推荐
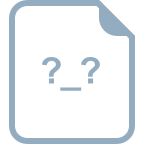

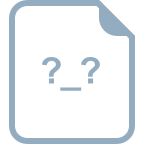
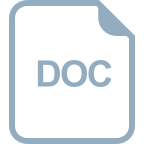
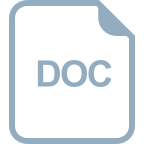

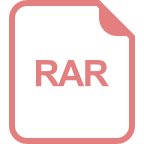
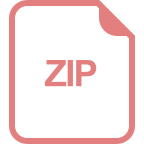
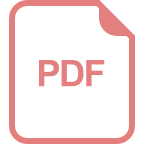
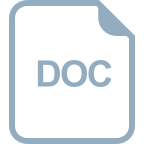
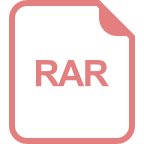
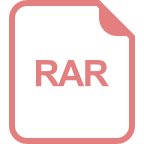
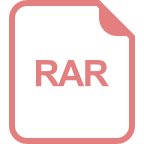
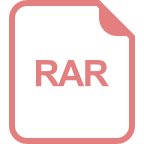
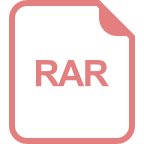
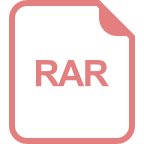
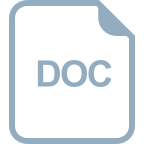