c++ 写一个消息订阅器class,根据消息类型type,绑定对应函数std::function,再有内部的线程对消息type进行分发到对应的函数
时间: 2024-11-06 09:16:21 浏览: 20
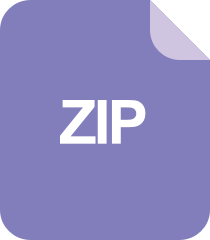
cpp代码-std::bind & function
在C++中,你可以创建一个名为`MessageSubscriber`的类,用于订阅不同类型的消息并调用对应的函数。这里是一个简单的例子:
```cpp
#include <iostream>
#include <thread>
#include <map>
#include <functional>
class MessageSubscriber {
public:
// 增加消息订阅
template<typename Func, typename T>
void subscribe(const std::string& type, Func&& func) {
message_map_[type] = std::bind(func, this);
}
// 发布消息并分发到对应函数
void publish(T message, const std::string& type) {
if (message_map_.find(type) != message_map_.end()) {
auto bound_func = message_map_[type];
std::invoke(bound_func, message); // 使用std::invoke避免捕获this
}
}
private:
std::map<std::string, std::function<void(T)>> message_map_;
};
// 示例:订阅和发布消息
int main() {
MessageSubscriber subscriber;
// 订阅消息类型为"hello"
subscriber.subscribe<std::function<void(int)>,"hello", [](int num) { std::cout << "Received hello message with number: " << num << std::endl; });
// 发布消息
int message = 123;
subscriber.publish(message, "hello");
return 0;
}
```
在这个例子中,`subscribe`方法接受一个类型参数`T`和一个函数模板`Func`,然后将`func`绑定到特定的消息类型上。当`publish`方法接收到相应类型的消息时,会从映射中找到相应的函数并执行。
注意,为了防止回调函数修改`subscriber`实例的成员,我们在`publish`中使用了`std::invoke`,而不是直接传递`this`给`bound_func`。
阅读全文
相关推荐
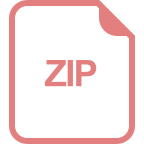
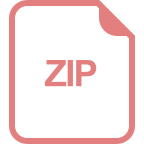

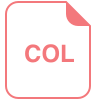
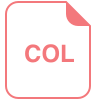
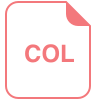
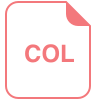
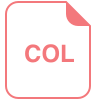
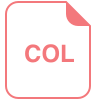
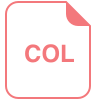
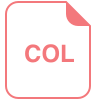
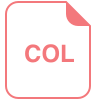
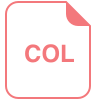
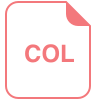
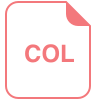
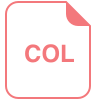
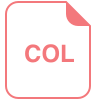
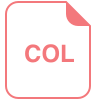