实现一个双向循环链表,数据域是int型值,并完成如下API编写 1,双向循环链表的创建list_create 2,双向循环链表的节点插入list_insert 3,根据数据域从小到大实现双向循环链表的排序list_sort
时间: 2024-10-13 18:02:52 浏览: 32
实现一个双向循环链表,首先我们需要定义一个Node结构体,包含整数值和指向前后节点的指针。然后可以按照以下步骤设计:
```c
// 定义双向循环链表节点
typedef struct Node {
int value;
struct Node* prev;
struct Node* next;
} Node;
// 双向循环链表结构体
typedef struct DoublyCircularList {
Node* head;
Node* tail;
} DoublyCircularList;
// 创建双向循环链表(list_create)
DoublyCircularList* list_create() {
DoublyCircularList* list = (DoublyCircularList*)malloc(sizeof(DoublyCircularList));
if (list) {
list->head = list->tail = NULL;
}
return list;
}
// 插入节点到链表(list_insert)
void list_insert(DoublyCircularList* list, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode) {
newNode->value = value;
newNode->prev = list->tail;
newNode->next = list->head;
if (list->head != NULL) {
list->head->prev = newNode;
}
if (list->tail != NULL) {
list->tail->next = newNode;
}
list->head = newNode;
list->tail = newNode;
} else {
printf("Memory allocation failed for new node.\n");
}
}
// 根据数据域从小到大排序链表(list_sort)
void list_sort(DoublyCircularList* list) {
if (list->head == NULL || list->head == list->tail) {
return; // 空链表或单元素链表无需排序
}
// 使用插入排序,遍历链表并比较相邻节点
Node* current = list->head;
while (current != list->tail) {
Node* temp = current->prev;
while (temp != list->head && temp->value > current->value) {
// 交换节点位置
int tempValue = temp->value;
temp->value = current->value;
current->value = tempValue;
temp = temp->prev;
}
current = current->next;
}
}
```
阅读全文
相关推荐
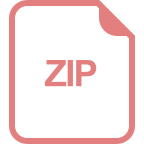
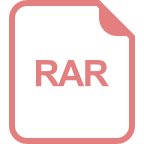
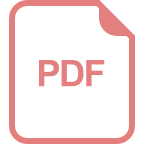















