创意题]设计Student类,包括属性“学号”、“姓名”和3门课程 math、english和java的成绩,包括的方法有计算3门课程的“总分”、“平均分”、“最高分”及“最低分”。创建班级中期末考试成绩单。同时在程序中输出你的姓名-班级-学号-程序运行的日期。java
时间: 2024-11-06 20:14:34 浏览: 32
首先,我们可以在Java中设计一个名为`Student`的类,包含所需的属性和方法:
```java
import java.time.LocalDate;
public class Student {
private String id; // 学号
private String name; // 姓名
private int mathScore;
private int englishScore;
private int javaScore;
public Student(String id, String name, int math, int english, int java) {
this.id = id;
this.name = name;
this.mathScore = math;
this.englishScore = english;
this.javaScore = java;
}
// 计算总分
public int calculateTotalScore() {
return mathScore + englishScore + javaScore;
}
// 计算平均分
public double calculateAverageScore() {
return (double) calculateTotalScore() / 3;
}
// 计算最高分
public int findMaxScore() {
return Math.max(mathScore, Math.max(englishScore, javaScore));
}
// 计算最低分
public int findMinScore() {
return Math.min(mathScore, Math.min(englishScore, javaScore));
}
// 创建中期末考试成绩单
public void createReport() {
LocalDate currentDate = LocalDate.now();
System.out.println("姓名: " + name + ", 班级: [自定义班级名], 学号: " + id);
System.out.println("当前日期: " + currentDate);
System.out.println("数学成绩: " + mathScore + ", 英语成绩: " + englishScore + ", Java成绩: " + javaScore);
System.out.println("总分: " + calculateTotalScore());
System.out.println("平均分: " + calculateAverageScore());
System.out.println("最高分: " + findMaxScore());
System.out.println("最低分: " + findMinScore());
}
}
```
在上述代码中,`createReport()`方法会打印出学生的个人信息以及他们的分数统计结果。你可以根据需要替换"[自定义班级名]"为实际的班级名称。
阅读全文
相关推荐
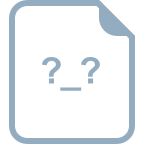
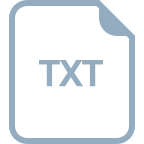
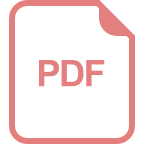














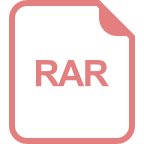