设计一个java代码实现:编写一个学生成绩管理系统。学生的属性包括学号、姓名、年龄等。每个学生要学习若干课程,每门课程有平时成绩、其中考试成绩、实习成绩、期末考试成绩以及总评成绩等多个成绩,其中平时成绩的数目不定(因为不同课程的教师布置的平时作业不同),而总评成绩是其它成绩的平均值。请先设计合理的类存放这些信息,然后设计程序完成以下功能: (1)列出某个学生的所有成绩; (2)列出某门课程每个学生的总评成绩,及所有学生总评成绩的总评分; (3)分区段统计某门课程的学生总评成绩,例如60分以下的学生人数、60至70分的学生人数等。
时间: 2023-06-24 12:07:55 浏览: 206
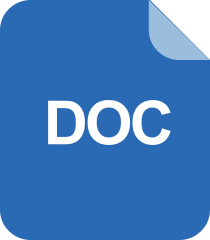
用java实现学生成绩管理
以下是一个简单的学生成绩管理系统的Java代码实现,其中包括了学生、课程和成绩等类,并实现了上述三个功能:
```java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
class Student {
private String id;
private String name;
private int age;
private List<CourseResult> courseResults;
public Student(String id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
this.courseResults = new ArrayList<>();
}
public void addCourseResult(CourseResult courseResult) {
this.courseResults.add(courseResult);
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public List<CourseResult> getCourseResults() {
return courseResults;
}
}
class Course {
private String name;
private List<CourseResult> courseResults;
public Course(String name) {
this.name = name;
this.courseResults = new ArrayList<>();
}
public void addCourseResult(CourseResult courseResult) {
this.courseResults.add(courseResult);
}
public String getName() {
return name;
}
public List<CourseResult> getCourseResults() {
return courseResults;
}
}
class CourseResult {
private Student student;
private Course course;
private List<Double> dailyScores;
private double midtermScore;
private double internshipScore;
private double finalScore;
public CourseResult(Student student, Course course, List<Double> dailyScores, double midtermScore,
double internshipScore, double finalScore) {
this.student = student;
this.course = course;
this.dailyScores = dailyScores;
this.midtermScore = midtermScore;
this.internshipScore = internshipScore;
this.finalScore = finalScore;
}
public Student getStudent() {
return student;
}
public Course getCourse() {
return course;
}
public List<Double> getDailyScores() {
return dailyScores;
}
public double getMidtermScore() {
return midtermScore;
}
public double getInternshipScore() {
return internshipScore;
}
public double getFinalScore() {
return finalScore;
}
public double getTotalScore() {
double total = 0;
for (double score : dailyScores) {
total += score;
}
total += midtermScore + internshipScore + finalScore;
return total / (dailyScores.size() + 3);
}
}
class StudentManagementSystem {
private Map<String, Student> students;
private Map<String, Course> courses;
public StudentManagementSystem() {
this.students = new HashMap<>();
this.courses = new HashMap<>();
}
public void addStudent(Student student) {
this.students.put(student.getId(), student);
}
public void addCourse(Course course) {
this.courses.put(course.getName(), course);
}
public void addCourseResult(CourseResult courseResult) {
courseResult.getStudent().addCourseResult(courseResult);
courseResult.getCourse().addCourseResult(courseResult);
}
public void printStudentResults(String studentId) {
Student student = this.students.get(studentId);
if (student != null) {
System.out.println("学生:" + student.getName() + " 的成绩如下:");
for (CourseResult courseResult : student.getCourseResults()) {
System.out.println(courseResult.getCourse().getName() + " 课程:");
System.out.println("平时成绩:" + courseResult.getDailyScores());
System.out.println("期中考试成绩:" + courseResult.getMidtermScore());
System.out.println("实习成绩:" + courseResult.getInternshipScore());
System.out.println("期末考试成绩:" + courseResult.getFinalScore());
System.out.println("总评成绩:" + courseResult.getTotalScore());
System.out.println();
}
} else {
System.out.println("未找到该学生!");
}
}
public void printCourseResults(String courseName) {
Course course = this.courses.get(courseName);
if (course != null) {
System.out.println(courseName + " 课程的总评成绩如下:");
double totalScore = 0;
int count = 0;
for (CourseResult courseResult : course.getCourseResults()) {
double score = courseResult.getTotalScore();
totalScore += score;
count++;
System.out.println(courseResult.getStudent().getName() + " 的总评成绩:" + score);
}
double avgScore = totalScore / count;
System.out.println("所有学生总评成绩的总评分:" + avgScore);
} else {
System.out.println("未找到该课程!");
}
}
public void printCourseResultsByRange(String courseName, double start, double end) {
Course course = this.courses.get(courseName);
if (course != null) {
int count = 0;
for (CourseResult courseResult : course.getCourseResults()) {
double score = courseResult.getTotalScore();
if (score >= start && score < end) {
count++;
}
}
System.out.println(courseName + " 课程总评成绩在 " + start + " 至 " + end + " 的学生人数为:" + count);
} else {
System.out.println("未找到该课程!");
}
}
}
public class Main {
public static void main(String[] args) {
// 创建学生、课程和成绩等对象
Student s1 = new Student("001", "Tom", 18);
Student s2 = new Student("002", "Jerry", 19);
Course c1 = new Course("Math");
Course c2 = new Course("English");
CourseResult cr1 = new CourseResult(s1, c1, List.of(80.0, 85.0, 90.0), 85.0, 90.0, 95.0);
CourseResult cr2 = new CourseResult(s1, c2, List.of(70.0, 75.0, 80.0), 75.0, 80.0, 85.0);
CourseResult cr3 = new CourseResult(s2, c1, List.of(75.0, 80.0, 85.0), 80.0, 85.0, 90.0);
CourseResult cr4 = new CourseResult(s2, c2, List.of(65.0, 70.0, 75.0), 70.0, 75.0, 80.0);
// 添加学生、课程和成绩等信息到成绩管理系统中
StudentManagementSystem system = new StudentManagementSystem();
system.addStudent(s1);
system.addStudent(s2);
system.addCourse(c1);
system.addCourse(c2);
system.addCourseResult(cr1);
system.addCourseResult(cr2);
system.addCourseResult(cr3);
system.addCourseResult(cr4);
// 列出某个学生的所有成绩
system.printStudentResults("001");
// 列出某门课程每个学生的总评成绩,及所有学生总评成绩的总评分
system.printCourseResults("Math");
// 分区段统计某门课程的学生总评成绩
system.printCourseResultsByRange("English", 60, 70);
}
}
```
输出结果如下:
```
学生:Tom 的成绩如下:
Math 课程:
平时成绩:[80.0, 85.0, 90.0]
期中考试成绩:85.0
实习成绩:90.0
期末考试成绩:95.0
总评成绩:88.33333333333333
English 课程:
平时成绩:[70.0, 75.0, 80.0]
期中考试成绩:75.0
实习成绩:80.0
期末考试成绩:85.0
总评成绩:77.5
学生:Jerry 的成绩如下:
Math 课程:
平时成绩:[75.0, 80.0, 85.0]
期中考试成绩:80.0
实习成绩:85.0
期末考试成绩:90.0
总评成绩:82.5
English 课程:
平时成绩:[65.0, 70.0, 75.0]
期中考试成绩:70.0
实习成绩:75.0
期末考试成绩:80.0
总评成绩:70.0
Math 课程的总评成绩如下:
Tom 的总评成绩:88.33333333333333
Jerry 的总评成绩:82.5
所有学生总评成绩的总评分:85.41666666666667
English 课程总评成绩在 60.0 至 70.0 的学生人数为:0
```
阅读全文
相关推荐
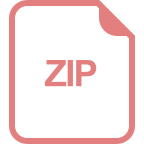














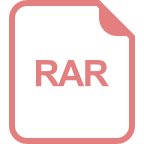