编写代码使得可以通过上下左右键的输入来控制Unity中GameObject的移动
时间: 2023-03-24 09:02:49 浏览: 70
您好,可以使用以下代码实现通过上下左右键的输入来控制Unity中GameObject的移动:
```
using UnityEngine;
public class MoveObject : MonoBehaviour
{
public float speed = 10.f;
void Update()
{
float horizontalInput = Input.GetAxis("Horizontal");
float verticalInput = Input.GetAxis("Vertical");
transform.Translate(new Vector3(horizontalInput, , verticalInput) * speed * Time.deltaTime);
}
}
```
其中,speed 变量可以控制移动速度。通过获取输入的 horizontalInput 和 verticalInput,使用 Translate 方法来实现移动。
相关问题
编写代码使得可以通过上下左右键的输入来控制Unity中GameObject的移动,并添加详细的注释
好的,以下是代码和注释:
// 定义一个变量来存储GameObject对象
GameObject player;
void Start()
{
// 获取场景中名为"Player"的GameObject对象
player = GameObject.Find("Player");
}
void Update()
{
// 获取键盘输入的方向键值
float horizontal = Input.GetAxis("Horizontal");
float vertical = Input.GetAxis("Vertical");
// 计算移动向量
Vector3 moveDirection = new Vector3(horizontal, , vertical);
// 将移动向量转换为世界坐标系下的向量
moveDirection = transform.TransformDirection(moveDirection);
// 设置移动速度
moveDirection *= 5;
// 移动GameObject对象
player.transform.Translate(moveDirection * Time.deltaTime);
}
// 注释:
// 1. 定义一个变量来存储GameObject对象
// 2. 在Start函数中获取场景中名为"Player"的GameObject对象
// 3. 在Update函数中获取键盘输入的方向键值
// 4. 计算移动向量,并将其转换为世界坐标系下的向量
// 5. 设置移动速度
// 6. 移动GameObject对象
编写代码用于移动unity中的GameObject
好的,我可以回答这个问题。要移动Unity中的GameObject,可以使用Transform组件的position属性来改变其位置。以下是一个示例代码:
```csharp
using UnityEngine;
public class MoveObject : MonoBehaviour
{
public float speed = 5f; // 移动速度
void Update()
{
// 获取当前位置
Vector3 pos = transform.position;
// 在x轴上移动
pos.x += speed * Time.deltaTime;
// 更新位置
transform.position = pos;
}
}
```
在这个示例中,我们定义了一个MoveObject类,它继承自MonoBehaviour。在Update方法中,我们获取了GameObject的当前位置,然后在x轴上移动一定距离,最后更新位置。你可以根据需要修改移动的速度和方向。
相关推荐
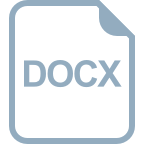
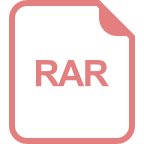
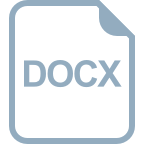












