Linux下的c语言图书管理系统
时间: 2024-02-07 11:41:03 浏览: 25
在Linux系统下,可以使用C语言编写一个简单的图书管理系统。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
struct book {
int id;
char title[50];
char author[50];
int year;
};
struct book books[MAX_BOOKS];
int num_books = 0;
void add_book() {
struct book new_book;
printf("Enter book ID: ");
scanf("%d", &new_book.id);
printf("Enter book title: ");
scanf("%s", new_book.title);
printf("Enter book author: ");
scanf("%s", new_book.author);
printf("Enter year of publication: ");
scanf("%d", &new_book.year);
books[num_books] = new_book;
num_books++;
printf("Book added successfully!\n");
}
void view_books() {
printf("ID\tTitle\tAuthor\tYear\n");
for (int i = 0; i < num_books; i++) {
struct book current_book = books[i];
printf("%d\t%s\t%s\t%d\n", current_book.id, current_book.title,
current_book.author, current_book.year);
}
}
void search_books() {
char search_term[50];
printf("Enter search term: ");
scanf("%s", search_term);
printf("ID\tTitle\tAuthor\tYear\n");
for (int i = 0; i < num_books; i++) {
struct book current_book = books[i];
if (strstr(current_book.title, search_term) != NULL || strstr(current_book.author, search_term) != NULL) {
printf("%d\t%s\t%s\t%d\n", current_book.id, current_book.title,
current_book.author, current_book.year);
}
}
}
int main() {
int choice;
while (1) {
printf("\n1. Add book\n");
printf("2. View books\n");
printf("3. Search books\n");
printf("4. Quit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book();
break;
case 2:
view_books();
break;
case 3:
search_books();
break;
case 4:
exit(0);
default:
printf("Invalid choice!\n");
}
}
return 0;
}
```
这个程序定义了一个结构体 `book` 表示一本书,每本书有一个ID、标题、作者和出版年份。程序使用一个数组 `books` 来存储所有的书籍,并提供了三个基本操作:添加书籍、查看所有书籍和搜索书籍。可以通过在终端中运行程序来使用这些操作。
相关推荐
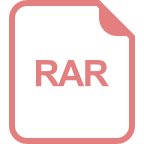
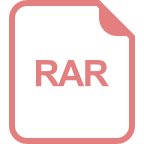















